Leetcode 1637: Widest Vertical Area Between Two Points Containing No Points
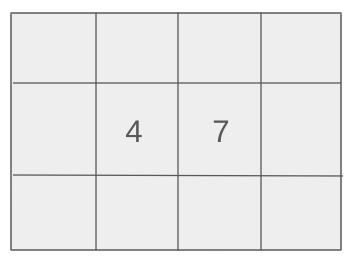
Given n points on a 2D plane, where points[i] = [xi, yi], return the widest vertical area between two points such that no points are inside the area. A vertical area is defined as a fixed-width region that extends infinitely along the y-axis. The widest vertical area is the one with the maximum width. Note that points on the edge of the vertical area are not considered inside.
Problem
Approach
Steps
Complexity
Input: The input consists of n points on a 2D plane, each represented as a pair of integers, [xi, yi], where xi and yi are the coordinates of the points.
Example: points = [[1, 4], [3, 1], [9, 0], [4, 6], [7, 2]]
Constraints:
• 2 <= n <= 10^5
• 0 <= xi, yi <= 10^9
Output: The output is the width of the widest vertical area between two points such that no points lie within the area.
Example: Output: 5
Constraints:
• The width will be a non-negative integer.
Goal: The goal is to find the widest vertical area between two points where no points are inside the area.
Steps:
• Sort the points based on their x-coordinates.
• Compute the horizontal distance between consecutive points in the sorted list.
• Return the maximum distance between consecutive points as the result.
Goal: The number of points is at least 2 and can go up to 100,000. The x and y values of the points are non-negative integers and can be as large as 10^9.
Steps:
• 2 <= n <= 100,000
• 0 <= xi, yi <= 10^9
Assumptions:
• There will always be at least two points in the array.
• Input: points = [[12, 5], [8, 7], [15, 10], [9, 6]]
• Explanation: The points are first sorted by their x-coordinate: [[8, 7], [9, 6], [12, 5], [15, 10]]. The distances between consecutive points are 1, 3, and 3, so the maximum width of the vertical area is 7.
Approach: We will approach this problem by sorting the points based on their x-coordinates and then calculating the horizontal distances between consecutive points. The maximum distance will be the answer.
Observations:
• Sorting the points by their x-coordinate will allow us to easily compute the distances between adjacent points.
• Sorting the points first is the key step, as it ensures we are considering the widest possible vertical area between points.
Steps:
• Sort the points by their x-coordinate.
• Iterate through the sorted list of points and calculate the distance between each pair of consecutive points.
• Track the maximum distance and return it as the result.
Empty Inputs:
• The problem guarantees that there will always be at least two points, so there is no need to handle empty inputs.
Large Inputs:
• For large inputs, the solution should still be efficient with time complexity O(n log n) due to the sorting step.
Special Values:
• The points may have large coordinates up to 10^9, but the solution will handle this without issue as long as the points are sorted first.
Constraints:
• The solution needs to handle arrays with up to 100,000 points efficiently.
int maxWidthOfVerticalArea(vector<vector<int>>& pts) {
sort(pts.begin(), pts.end());
int res =0;
for(int i = 1; i < pts.size(); i++)
res = max(res, pts[i][0] - pts[i - 1][0]);
return res;
}
};
1 : Method Definition
int maxWidthOfVerticalArea(vector<vector<int>>& pts) {
Define the method 'maxWidthOfVerticalArea' that takes a 2D vector of points 'pts' as input.
2 : Sorting
sort(pts.begin(), pts.end());
Sort the points based on their x-coordinates to prepare for calculating the maximum vertical width.
3 : Variable Initialization
int res =0;
Initialize the variable 'res' to store the maximum vertical width found during the iteration.
4 : Loop Constructs
for(int i = 1; i < pts.size(); i++)
Start a loop that iterates over the points from the second element to the last.
5 : Mathematical Operations
res = max(res, pts[i][0] - pts[i - 1][0]);
For each pair of consecutive points, calculate the width (difference between the x-coordinates) and update 'res' if a larger width is found.
6 : Return Statement
return res;
Return the maximum width found during the loop.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is O(n log n) due to the sorting step.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) for storing the sorted list of points.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus