Leetcode 1598: Crawler Log Folder
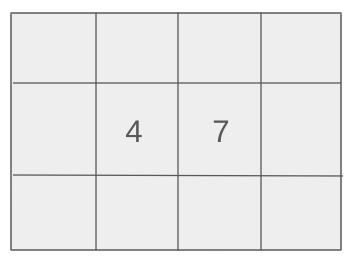
You are working with a file system that logs folder change operations. The file system starts in the main folder, and the operations allow the user to move to a parent folder, remain in the current folder, or navigate to a child folder. Given a list of operations, your task is to determine the minimum number of operations required to return to the main folder after performing all the operations.
Problem
Approach
Steps
Complexity
Input: You are given a list of strings where each string represents a folder operation. Operations include moving to a parent folder ('../'), staying in the current folder ('./'), or moving to a child folder ('x/').
Example: Input: logs = ['folder1/', 'folder2/', '../', 'folder21/', './']
Constraints:
• 1 <= logs.length <= 1000
• 2 <= logs[i].length <= 10
• logs[i] contains lowercase English letters, digits, '.', and '/'
• logs[i] follows the described format
Output: Return the minimum number of operations required to return to the main folder after the changes are applied.
Example: Output: 2
Constraints:
• The returned number is a non-negative integer.
Goal: The goal is to find the minimum number of operations to go back to the main folder.
Steps:
• 1. Initialize a counter to keep track of the current folder level, starting at 0 (main folder).
• 2. For each operation, adjust the counter based on the operation type ('../' decreases level, 'x/' increases level, './' leaves the level unchanged).
• 3. After processing all operations, the counter will indicate the number of operations needed to return to the main folder.
Goal: The input list contains folder operations that follow a specified format, and the size of the list is manageable within the problem's constraints.
Steps:
• 1 <= logs.length <= 1000
• Each folder operation string has a length between 2 and 10 characters.
Assumptions:
• The folder operations are guaranteed to follow the specified format.
• Input: Input: logs = ['folder1/', 'folder2/', '../', 'folder21/', './']
• Explanation: In this case, we move to 'folder1', then to 'folder2', go back to the main folder using '../', move to 'folder21', and stay in the current folder. To return to the main folder, we need to move back twice using '../', hence the output is 2.
• Input: Input: logs = ['folder1/', 'folder2/', './', 'folder3/', '../', 'folder31/']
• Explanation: After moving through various folders, we must return 3 levels up to get back to the main folder. Thus, the output is 3.
• Input: Input: logs = ['folder1/', '../', '../', '../']
• Explanation: In this case, after moving to 'folder1', we use '../' three times to get back to the main folder, and since we're already in the main folder, the output is 0.
Approach: The approach involves simulating the folder change operations, adjusting the folder level accordingly. We use a simple counter to track the number of steps required to return to the main folder.
Observations:
• Each operation can either increase, decrease, or leave the folder level unchanged.
• We don't need to simulate every individual operation, but rather just track the folder depth.
• By keeping track of the folder level, we can directly compute the minimum number of operations to return to the main folder.
Steps:
• 1. Initialize a counter `level` to 0, representing the main folder.
• 2. Iterate over the logs and update `level` according to the operation type: decrease for '../', increase for 'x/', and do nothing for './'.
• 3. Return the final value of `level` as the number of operations required to return to the main folder.
Empty Inputs:
• If no operations are provided, return 0.
Large Inputs:
• The problem can handle up to 1000 operations, so the solution should efficiently handle large input sizes.
Special Values:
• Consider cases where all operations are './', which would mean no movement and thus 0 operations to return.
Constraints:
• Ensure the solution works within the constraints, especially the maximum size of the input list.
static int minOperations(vector<string>& logs) {
int level=0;
for(auto& dir: logs){
if (dir=="../")
level-=(level>0);
else if (dir!="./")
level++;
// cout<<level<<endl;
}
return level;
}
1 : Function Definition
static int minOperations(vector<string>& logs) {
This is the function signature. It defines a static function 'minOperations' that takes a reference to a vector of strings, 'logs', representing the directory operations.
2 : Variable Initialization
int level=0;
Here, a variable 'level' is initialized to 0. This variable will be used to track the current level in the directory structure.
3 : Loop
for(auto& dir: logs){
This 'for' loop iterates over each directory operation in the 'logs' vector.
4 : Condition Check
if (dir=="../")
This condition checks if the current operation is moving up one directory ('../').
5 : Level Adjustment
level-=(level>0);
If the 'dir' is '../', the level is decremented by 1, but only if the current level is greater than 0, ensuring that it doesn't go below 0.
6 : Condition Check
else if (dir!="./")
This condition checks if the directory operation is not './', i.e., the operation is a valid directory movement (entering a new directory).
7 : Level Adjustment
level++;
If the directory operation is not './' (i.e., a new directory is entered), the level is incremented by 1.
8 : Return Statement
return level;
After all operations have been processed, the final 'level' is returned, indicating the final position in the directory structure.
Best Case: O(n) - If all operations are './', no level changes are needed.
Average Case: O(n) - We always iterate over the input list once.
Worst Case: O(n) - In the worst case, we may need to process all operations.
Description: The time complexity is O(n), where n is the length of the logs list.
Best Case: O(1) - The space complexity remains constant regardless of the input size.
Worst Case: O(1) - We only need a few variables to track the current folder level.
Description: The space complexity is O(1), as we are only using a fixed amount of memory to store the folder level.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus