Leetcode 1578: Minimum Time to Make Rope Colorful
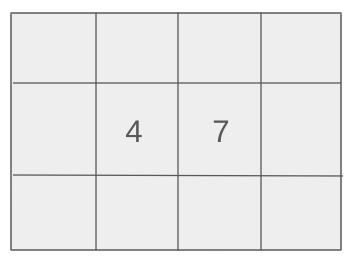
Alice has a rope with balloons arranged in a sequence where each balloon has a color. She wants the rope to be colorful, meaning no two consecutive balloons should have the same color. Bob is tasked with helping Alice by removing some balloons. Each balloon has an associated cost, and Bob needs to minimize the total time spent removing the balloons to achieve the desired colorful sequence. Return the minimum time Bob needs to make the rope colorful.
Problem
Approach
Steps
Complexity
Input: You are given a string 'colors' representing the colors of the balloons, where each character corresponds to a balloon, and an array 'neededTime' representing the time in seconds it takes to remove the corresponding balloon.
Example: Input: colors = 'abcac', neededTime = [1, 2, 3, 4, 5]
Constraints:
• 1 <= n <= 10^5
• 1 <= neededTime[i] <= 10^4
• colors contains only lowercase English letters.
Output: Return an integer representing the minimum time Bob needs to remove the balloons to make the rope colorful.
Example: Output: 3
Constraints:
Goal: The goal is to minimize the total time by removing the minimum cost balloons that are consecutive and of the same color.
Steps:
• 1. Iterate over the balloons while checking for consecutive ones with the same color.
• 2. For each consecutive pair, keep the balloon with the higher removal cost and remove the one with the lower cost.
• 3. Add the removal time of the lower-cost balloon to the total removal time.
Goal: The number of balloons can be large, so the solution must be efficient.
Steps:
• n is the length of the 'colors' string and 'neededTime' array, and n can be as large as 100,000.
• Each time value in 'neededTime' is between 1 and 10,000.
Assumptions:
• The input size is large, so the solution must be optimized to handle up to 100,000 balloons efficiently.
• Each balloon can only be removed once.
• Input: Example 1: colors = 'abcac', neededTime = [1, 2, 3, 4, 5]
• Explanation: In this case, there are two consecutive balloons with the color 'a' at indices 0 and 4. The balloon at index 0 has a removal cost of 1, and the balloon at index 4 has a removal cost of 5. We keep the one with the higher cost (index 4) and remove the balloon at index 0, resulting in a total removal time of 3.
• Input: Example 2: colors = 'abc', neededTime = [1, 2, 3]
• Explanation: There are no consecutive balloons with the same color, so no removal is necessary. The total removal time is 0.
• Input: Example 3: colors = 'aabaa', neededTime = [1, 2, 3, 4, 1]
• Explanation: The consecutive balloons of the same color are at indices 0 and 4 (both 'a'). The balloon at index 0 has a removal cost of 1, and the balloon at index 4 has a removal cost of 1. We remove both of them, resulting in a total removal time of 2.
Approach: To solve this problem, we need to efficiently handle consecutive balloons of the same color. The approach involves iterating through the string of colors, checking for consecutive colors, and removing the cheaper balloon while keeping track of the total time.
Observations:
• The main challenge is to find and handle consecutive balloons of the same color.
• We need to minimize the total removal time by removing the balloon with the lower removal cost in each consecutive sequence of same-colored balloons.
• Using a greedy approach works well here by removing the lowest-cost balloon in each group of consecutive same-colored balloons.
Steps:
• 1. Initialize a variable to store the total removal time.
• 2. Iterate over the colors array, comparing consecutive balloons of the same color.
• 3. For each group of consecutive balloons, calculate the total removal time by removing the balloon with the smaller removal cost.
• 4. Return the total removal time after processing the entire string.
Empty Inputs:
• There are no empty inputs because the length of 'colors' is at least 1.
Large Inputs:
• The solution should efficiently handle inputs where the length of 'colors' is up to 100,000.
Special Values:
• The 'colors' string may contain only one color, in which case all consecutive balloons will be the same, and we will remove all but the one with the highest cost.
Constraints:
• The solution needs to handle the maximum input size efficiently.
int minCost(string colors, vector<int>& cost) {
int res = 0, sum, mx;
int i = 1, n = cost.size();
while(i < n) {
int e = i;
sum = cost[e - 1];
mx = cost[e - 1];
while(e < n && colors[e] == colors[e-1]) {
sum += cost[e];
mx = max(mx, cost[e]);
e++;
}
res += (sum-mx);
i = e + 1;
}
return res;
}
1 : Function Definition
int minCost(string colors, vector<int>& cost) {
Define the function `minCost`, which takes a string `colors` representing house colors and a vector `cost` representing the cost to paint each house.
2 : Variable Initialization
int res = 0, sum, mx;
Initialize `res` to store the result (total minimum cost), `sum` to store the sum of costs for consecutive houses with the same color, and `mx` to store the maximum cost in the same segment.
3 : Variable Initialization
int i = 1, n = cost.size();
Initialize `i` to 1 (the starting index for the loop) and `n` to the size of the `cost` vector (number of houses).
4 : While Loop - Outer Loop
while(i < n) {
Start the outer loop to iterate through each house in the array.
5 : Variable Initialization - Inner Loop
int e = i;
Initialize `e` to `i` (the start of a new segment of consecutive houses with the same color).
6 : Assigning Sum and Max
sum = cost[e - 1];
Set `sum` to the cost of the previous house (`cost[e - 1]`), as `e` points to the current house.
7 : Assigning Max
mx = cost[e - 1];
Set `mx` to the same value as `sum`, which is the cost of the previous house.
8 : While Loop - Inner Loop
while(e < n && colors[e] == colors[e-1]) {
Start the inner loop to check for consecutive houses with the same color. If `colors[e]` is the same as `colors[e-1]`, continue iterating.
9 : Updating Sum
sum += cost[e];
Add the cost of the current house to `sum` for the consecutive houses with the same color.
10 : Updating Max
mx = max(mx, cost[e]);
Update `mx` to the maximum cost encountered in the current segment of consecutive houses.
11 : Incrementing Inner Loop Index
e++;
Increment `e` to move to the next house in the segment.
12 : Update Result
res += (sum - mx);
Update the result `res` by adding the difference between the total sum of costs for the segment and the maximum cost (as this is the cost we would avoid).
13 : Update Outer Loop Index
i = e + 1;
Set `i` to `e + 1` to move to the next new segment of houses with a different color.
14 : Return Result
return res;
Return the final result, which is the minimum cost to paint all the houses according to the given rules.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) since we only need to process each balloon once, where n is the length of the 'colors' array.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) since we only need a few variables to store intermediate results, and we are not using any additional data structures that scale with the input size.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus