Leetcode 1551: Minimum Operations to Make Array Equal
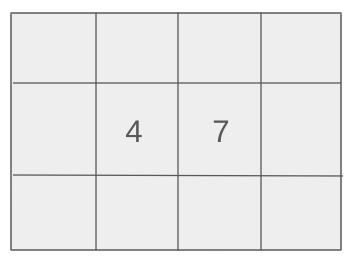
Given an integer n, create an array arr of length n where arr[i] = (2 * i) + 1. You can perform operations to make all elements in the array equal, with the goal of minimizing the number of operations.
Problem
Approach
Steps
Complexity
Input: The input consists of a single integer n, representing the length of the array.
Example: n = 4
Constraints:
• 1 <= n <= 10^4
Output: Return the minimum number of operations required to make all the elements in the array equal.
Example: Output: 4
Constraints:
• The operations should minimize the number of steps to equalize all elements.
Goal: The goal is to find the minimum number of operations required to make all elements equal.
Steps:
• 1. Calculate the target value (average of all elements).
• 2. Calculate how many operations are needed to move each element towards the target.
• 3. Perform operations to redistribute the values to achieve equality with the fewest steps.
Goal: The solution must handle input sizes efficiently, up to n = 10^4.
Steps:
• 1 <= n <= 10^4
Assumptions:
• The input n is always a positive integer within the specified bounds.
• Input: n = 4
• Explanation: The array starts as [1, 3, 5, 7]. The goal is to make all elements equal to 4 by minimizing the number of operations. The operations are performed as described to achieve equality in 4 steps.
• Input: n = 5
• Explanation: For n = 5, the array is [1, 3, 5, 7, 9]. The goal is to make all elements equal to 5, and it takes 6 operations to do so.
Approach: To minimize the number of operations, we calculate the target value (the average) and use efficient redistribution of values to achieve equality.
Observations:
• The values in the array are already in an increasing pattern, which makes the task of balancing the values easier.
• The solution should focus on redistributing the values towards the target value and minimizing the number of moves to balance the array.
Steps:
• 1. Calculate the target value, which is the average of the array.
• 2. For each element, calculate how many operations are needed to move it towards the target.
• 3. Use a greedy approach to balance the values, minimizing the number of operations.
Empty Inputs:
• The input array is never empty as n is always >= 1.
Large Inputs:
• The solution should handle large values of n (up to 10^4) efficiently.
Special Values:
• If n is 1, no operations are needed as the array already has a single element.
Constraints:
• Ensure the solution works for values of n up to 10^4.
int minOperations(int n) {
return n * n / 4;
}
1 : Function Declaration
int minOperations(int n) {
This is the function declaration where `minOperations` is defined to take an integer `n` as input and returns the minimum number of operations, calculated as `n * n / 4`.
2 : Return Statement
return n * n / 4;
The function returns the result of the mathematical expression `n * n / 4`, which is the calculated value based on the input `n`.
Best Case: O(1)
Average Case: O(1)
Worst Case: O(1)
Description: The solution involves calculating the target value and performing a few mathematical operations, so it is constant time.
Best Case: O(1)
Worst Case: O(1)
Description: The solution uses only a constant amount of space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus