Leetcode 1529: Minimum Suffix Flips
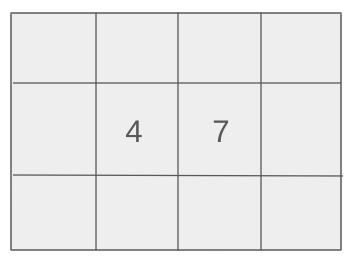
You are given a binary string
target
and another string s
initialized to all zeros. In one operation, you can flip all bits in the inclusive range starting from index i
to n-1
. The task is to determine the minimum number of operations needed to make s
equal to target
.Problem
Approach
Steps
Complexity
Input: A binary string `target` of length `n`.
Example: target = '10010'
Constraints:
• 1 <= n <= 10^5
• target[i] is either '0' or '1'.
Output: Return the minimum number of operations required to make `s` equal to `target`.
Example: For target = '10010', the output is 3.
Constraints:
• The output is the minimum number of operations required.
Goal: Minimize the number of operations by choosing the correct index `i` to flip bits.
Steps:
• Start with the string `s` initialized to all zeros.
• Iterate over the `target` string, flipping bits only when needed to match the target.
• Count the number of flip operations required.
Goal: Ensure the solution handles large input sizes efficiently.
Steps:
• 1 <= n <= 10^5
• target[i] is either '0' or '1'.
Assumptions:
• The string `s` is initially all zeros.
• Input: target = '10010'
• Explanation: We need to perform 3 operations to transform the string `s = '00000'` to the target `10010`.
• Input: target = '110'
• Explanation: 2 operations are enough to transform `s = '000'` to `110`.
Approach: The solution involves flipping the bits of `s` strategically to match `target` while minimizing the number of operations.
Observations:
• We only flip bits when the bit in `s` doesn't match `target`.
• We can track the changes in the string `s` while iterating through `target` and perform flips only when necessary.
Steps:
• Initialize `s` as all zeros.
• Iterate over `target` and compare each bit with the corresponding bit in `s`.
• Flip the bits from the current index to the end of the string whenever a mismatch is found.
• Count and return the total number of flips performed.
Empty Inputs:
• No empty string inputs as `n >= 1`.
Large Inputs:
• The solution should be efficient enough to handle up to 10^5 characters.
Special Values:
• If `target` is already equal to `s`, no flips are needed.
Constraints:
• The solution should work within the given time and space constraints.
int minFlips(string target) {
int flips = 0;
char status = '0';
for(int i = 0; i < target.size(); i++) {
if(status != target[i]) {
flips++;
status = status == '0'? '1':'0';
}
}
return flips;
}
1 : Function Declaration
int minFlips(string target) {
This line declares the function `minFlips`, which takes a string `target` and returns an integer representing the minimum number of flips needed to convert a binary string to match the `target` string.
2 : Initialize Flips Counter
int flips = 0;
The variable `flips` is initialized to zero. It will count the number of flips required to match the target binary string.
3 : Initialize Status
char status = '0';
The `status` variable is initialized to '0', representing the starting state of the binary string. This will be compared to each character in the `target` string to determine if a flip is required.
4 : Iterate Over Target String
for(int i = 0; i < target.size(); i++) {
This loop iterates through the `target` string, checking each character to see if a flip is required to match the `status`.
5 : Check for Flip Condition
if(status != target[i]) {
This condition checks if the current `status` (either '0' or '1') does not match the character at `target[i]`. If they don't match, it means a flip is needed.
6 : Increment Flip Counter
flips++;
If a flip is required, the `flips` counter is incremented.
7 : Toggle Status
status = status == '0'? '1':'0';
This line toggles the `status` variable. If the current `status` is '0', it changes to '1', and vice versa. This simulates the flipping process.
8 : Return Result
return flips;
The function returns the total number of flips (`flips`) required to convert the initial binary string (starting with '0') to match the target binary string.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The solution requires one pass through the string, resulting in a time complexity of O(n).
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) as we are only using a constant amount of extra space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus