Leetcode 1509: Minimum Difference Between Largest and Smallest Value in Three Moves
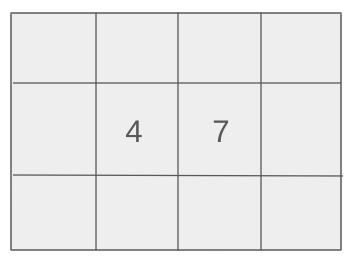
You are given an integer array. In one move, you can change any element of the array to any value. Perform at most three moves to minimize the difference between the largest and smallest values of the array.
Problem
Approach
Steps
Complexity
Input: The input consists of an integer array nums where each element can range from -10^9 to 10^9.
Example: nums = [4, 3, 5, 2]
Constraints:
• 1 <= nums.length <= 10^5
• -10^9 <= nums[i] <= 10^9
Output: Return the minimum difference between the maximum and minimum values in the array after performing at most three moves.
Example: 0
Constraints:
• Return the result as an integer.
Goal: The goal is to minimize the difference between the maximum and minimum values of the array after performing at most 3 moves.
Steps:
• Step 1: Sort the array to easily access the smallest and largest values.
• Step 2: Try changing the largest and smallest values to reduce the difference.
• Step 3: Return the minimum difference after performing at most three moves.
Goal: Ensure that the input array meets the constraints given in the problem description.
Steps:
• 1 <= nums.length <= 10^5
• -10^9 <= nums[i] <= 10^9
Assumptions:
• You are allowed to change any element to any other value.
• You can perform at most three moves.
• Input: nums = [4, 3, 5, 2]
• Explanation: After performing three moves, we can make all elements equal to 3, resulting in a minimum difference of 0.
• Input: nums = [1, 6, 8, 10, 15]
• Explanation: After performing three moves, the difference between the largest and smallest values is minimized to 1.
Approach: We need to minimize the difference between the largest and smallest values of the array by performing at most three moves.
Observations:
• Sorting the array helps in quickly accessing the smallest and largest values.
• Consider all possible ways to change the three largest and smallest values and check the resulting differences.
Steps:
• Step 1: Sort the array to get a clear view of the largest and smallest values.
• Step 2: Consider the effect of changing the largest and smallest values.
• Step 3: Return the smallest difference possible after making up to three moves.
Empty Inputs:
• The array should not be empty as per the problem constraints.
Large Inputs:
• Ensure the solution works efficiently even with large arrays (up to 100,000 elements).
Special Values:
• Handle arrays with a small number of elements (e.g., 2 or 3 elements).
Constraints:
• Ensure that no element exceeds the allowed range of values.
int minDifference(vector<int>& nums) {
int n = nums.size();
/* change one element to any other value in one move */
/* get min diff between max and min value after performing atmost 3 moves */
if(n < 5) return 0;
sort(nums.begin(), nums.end());
return min({nums[n - 1] - nums[3], nums[n - 2] - nums[2], nums[n - 3] - nums[1], nums[n - 4] - nums[0]});
}
1 : Function Definition
int minDifference(vector<int>& nums) {
Defines the `minDifference` function, which takes a vector `nums` as input and aims to minimize the difference between the maximum and minimum values after at most three changes.
2 : Variable Initialization
int n = nums.size();
Initializes a variable `n` to store the size of the vector `nums`. This is used to determine the number of elements in the array.
3 : Condition Check
if(n < 5) return 0;
Checks if the size of the array is less than 5. If it is, the function returns 0, as no moves are necessary in this case.
4 : Sorting
sort(nums.begin(), nums.end());
Sorts the vector `nums` in ascending order to facilitate easier calculations of the possible differences between elements after moves.
5 : Min Difference Calculation
return min({nums[n - 1] - nums[3], nums[n - 2] - nums[2], nums[n - 3] - nums[1], nums[n - 4] - nums[0]});
Calculates the minimum difference between the maximum and minimum values in the sorted array after performing at most three changes by evaluating specific differences.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is O(n log n) due to the sorting step.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) as we store the array.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus