Leetcode 1508: Range Sum of Sorted Subarray Sums
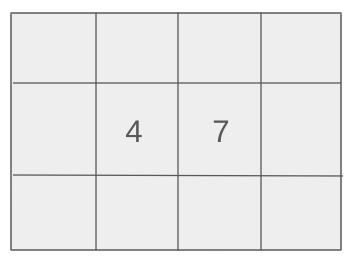
You are given an array of positive integers. Compute all possible subarray sums, sort them in non-decreasing order, and return the sum of elements from index left to right in the sorted array modulo 10^9 + 7.
Problem
Approach
Steps
Complexity
Input: You are given an array of size n, and you are asked to compute the sum of all non-empty subarrays, sort them, and then return a specific sum from the sorted array.
Example: nums = [1, 2, 3, 4], n = 4, left = 1, right = 5
Constraints:
• 1 <= nums.length <= 1000
• 1 <= nums[i] <= 100
• 1 <= left <= right <= n * (n + 1) / 2
Output: The output is a single integer, which is the sum of the elements from index left to right in the sorted list of all subarray sums, modulo 10^9 + 7.
Example: 13
Constraints:
• The output should be returned modulo 10^9 + 7.
Goal: The goal is to compute the sum of all subarrays, sort them, and compute the sum of the elements within the specified range.
Steps:
• Step 1: Generate all subarray sums by iterating over all pairs of indices in the array.
• Step 2: Sort the subarray sums in non-decreasing order.
• Step 3: Return the sum of the elements from index left to right in the sorted list, modulo 10^9 + 7.
Goal: The input array nums can have up to 1000 elements, and each element is a positive integer not exceeding 100.
Steps:
• 1 <= nums.length <= 1000
• 1 <= nums[i] <= 100
• 1 <= left <= right <= n * (n + 1) / 2
Assumptions:
• The input array will always be non-empty and consist of positive integers.
• Input: nums = [1, 2, 3, 4], n = 4, left = 1, right = 5
• Explanation: After sorting the subarray sums, the sum of elements from index 1 to 5 is 13.
• Input: nums = [1, 2, 3, 4], n = 4, left = 3, right = 4
• Explanation: After sorting the subarray sums, the sum of elements from index 3 to 4 is 6.
Approach: To solve this problem, we need to calculate all subarray sums, sort them, and then compute the sum of the elements in the sorted array for the specified range.
Observations:
• Sorting all subarray sums can be time-consuming, especially with large input sizes.
• Efficiently compute the subarray sums and handle sorting to avoid excessive time complexity.
Steps:
• Step 1: Iterate through the array to calculate all possible subarray sums.
• Step 2: Store these sums in an array and sort it.
• Step 3: Sum the elements in the specified range (left to right) and return the result modulo 10^9 + 7.
Empty Inputs:
• If the input array is empty, the result should be 0.
Large Inputs:
• Ensure the solution can handle the maximum array size efficiently.
Special Values:
• Consider cases where the array contains identical elements or where there are small subarrays.
Constraints:
• The input array should respect the given constraints.
int mod = (int) 1e9 + 7;
int rangeSum(vector<int>& nums, int n, int left, int right) {
// binary search
vector<int> ans;
for(int i = 0; i < n; i++) {
int sum = 0;
for(int j = i; j < n; j++) {
sum = (sum + nums[j]) % mod;
ans.push_back(sum);
}
}
sort(ans.begin(), ans.end());
int res = 0;
for(int i = left - 1; i < right; i++)
res = (res + ans[i]) % mod;
return res;
}
1 : Constant Initialization
int mod = (int) 1e9 + 7;
Initializes the constant `mod` with the value 1e9 + 7, which is used to take the modulo of the sums to avoid overflow.
2 : Function Definition
int rangeSum(vector<int>& nums, int n, int left, int right) {
Defines the `rangeSum` function which takes a vector `nums`, its size `n`, and two integers `left` and `right` to calculate the sum of subarrays between indices `left` and `right`.
3 : Variable Initialization
vector<int> ans;
Initializes an empty vector `ans` that will store the sums of all possible subarrays.
4 : Loop Initialization
for(int i = 0; i < n; i++) {
Starts a loop that iterates over all the elements of the input array `nums`.
5 : Variable Initialization
int sum = 0;
Initializes a variable `sum` to 0, which will store the sum of the current subarray.
6 : Nested Loop
for(int j = i; j < n; j++) {
Starts a nested loop that iterates through the elements of `nums`, starting from index `i`.
7 : Subarray Sum Calculation
sum = (sum + nums[j]) % mod;
Calculates the sum of the current subarray by adding `nums[j]` and taking the result modulo `mod`.
8 : Vector Update
ans.push_back(sum);
Pushes the current subarray sum to the vector `ans`.
9 : Sorting
sort(ans.begin(), ans.end());
Sorts the vector `ans` containing the subarray sums in ascending order.
10 : Variable Initialization
int res = 0;
Initializes `res` to 0, which will store the sum of the subarray sums in the specified range.
11 : Loop
for(int i = left - 1; i < right; i++)
Iterates over the sorted vector `ans` from the `left - 1` to `right - 1` to select the required subarray sums.
12 : Sum Calculation
res = (res + ans[i]) % mod;
Adds the current subarray sum to `res` and takes the result modulo `mod` to prevent overflow.
13 : Return
return res;
Returns the final result, which is the sum of subarray sums in the specified range [left, right], modulo 1e9 + 7.
Best Case: O(n^2 log n)
Average Case: O(n^2 log n)
Worst Case: O(n^2 log n)
Description: The time complexity is dominated by the sorting step, which is O(n^2 log n).
Best Case: O(n^2)
Worst Case: O(n^2)
Description: The space complexity is O(n^2) because we store all subarray sums.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus