Leetcode 1480: Running Sum of 1d Array
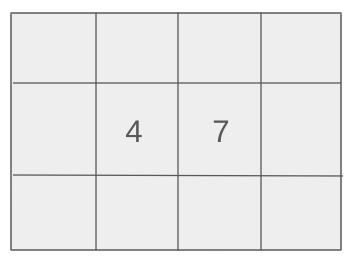
You are given an array of integers nums. Your task is to calculate the running sum of the array, where each element at index i represents the sum of all elements from index 0 to index i in the original array.
Problem
Approach
Steps
Complexity
Input: The input consists of a single array of integers nums.
Example: [4, 3, 2, 1]
Constraints:
• 1 <= nums.length <= 1000
• -10^6 <= nums[i] <= 10^6
Output: The output should be an array of integers representing the running sum of the input array.
Example: [4, 7, 9, 10]
Constraints:
• The length of the output array will be the same as the input array.
Goal: The goal is to compute the running sum by iterating through the input array and adding each element to the sum of previous elements.
Steps:
• Start by setting the running sum to the first element.
• Iterate through the rest of the elements, adding each element to the previous sum and storing the result at the current index.
Goal: The constraints ensure that the input array contains a manageable number of elements and values within the specified limits.
Steps:
• 1 <= nums.length <= 1000
• -10^6 <= nums[i] <= 10^6
Assumptions:
• The array will always have at least one element.
• The values in the array can be both positive and negative.
• Input: [4, 3, 2, 1]
• Explanation: Here, the running sum is calculated as follows: [4, 7, 9, 10].
Approach: To compute the running sum, iterate through the array, updating the current element by adding it to the sum of previous elements.
Observations:
• The problem requires an efficient solution since the array size can be as large as 1000.
• We can solve the problem in linear time by modifying the array in place.
Steps:
• Iterate through the array starting from index 1.
• For each index i, add nums[i] to nums[i-1] to accumulate the running sum.
• Return the modified array after the iteration.
Empty Inputs:
• If the array is empty, return an empty array.
Large Inputs:
• The solution should handle arrays of length up to 1000 efficiently.
Special Values:
• Handle cases where the array contains negative values.
Constraints:
• Ensure that the array length does not exceed the given limit.
vector<int> runningSum(vector<int>& nums) {
for(int i = 1; i < nums.size(); i++)
nums[i] += nums[i - 1];
return nums;
}
1 : Method
vector<int> runningSum(vector<int>& nums) {
This is the definition of the function `runningSum`, which takes an array `nums` by reference and returns an array of running sums.
2 : Loop
for(int i = 1; i < nums.size(); i++)
This for loop starts from index 1 and iterates through the `nums` array to compute the running sum for each element.
3 : Update
nums[i] += nums[i - 1];
In each iteration, the current element `nums[i]` is updated by adding the value of the previous element `nums[i - 1]` to it, thus building the running sum.
4 : Return
return nums;
Once the loop completes, the modified `nums` array, which now contains the running sums, is returned.
Best Case: O(n) where n is the length of the array.
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) since we only need to iterate over the array once.
Best Case: O(1)
Worst Case: O(1) if we modify the array in place.
Description: The space complexity is O(1) since we modify the array in place and do not require extra space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus