Leetcode 1464: Maximum Product of Two Elements in an Array
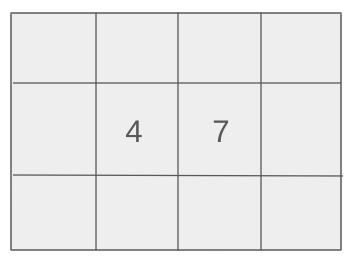
Given an array of integers, you need to find two distinct elements and calculate the product of their decremented values. Specifically, you are tasked with returning the maximum value of (nums[i] - 1) * (nums[j] - 1) for two different indices i and j in the array.
Problem
Approach
Steps
Complexity
Input: The input consists of a single array of integers. The length of the array will be at least 2. Each element of the array is a positive integer.
Example: Input: nums = [6, 3, 8, 2]
Constraints:
• 2 <= nums.length <= 500
• 1 <= nums[i] <= 1000
Output: The output is a single integer, representing the maximum value obtained by multiplying the decremented values of two distinct elements in the array.
Example: Output: 56
Constraints:
• The result should be the maximum possible value of (nums[i] - 1) * (nums[j] - 1), where i and j are distinct indices.
Goal: The goal is to find the two largest numbers in the array, subtract 1 from each, and multiply them to get the maximum product.
Steps:
• Identify the two largest numbers in the array.
• Decrement each of these numbers by 1.
• Multiply the decremented values and return the result as the output.
Goal: The problem has constraints that ensure the solution can be computed efficiently.
Steps:
• The array length will always be between 2 and 500.
• Each element in the array will be between 1 and 1000.
Assumptions:
• The input array will always contain at least two elements.
• The input values are valid and within the specified range.
• Input: Input: nums = [6, 3, 8, 2]
• Explanation: Output: 56. The two largest numbers are 8 and 6. After subtracting 1 from each, we get 7 and 5. Their product is 7 * 5 = 56.
• Input: Input: nums = [1, 5, 3, 9]
• Explanation: Output: 32. The two largest numbers are 9 and 5. After subtracting 1 from each, we get 8 and 4. Their product is 8 * 4 = 32.
Approach: To solve this problem, we can simply identify the two largest numbers in the array and compute their product after subtracting 1 from each of them.
Observations:
• We only need to find the two largest values in the array to maximize the result.
• Sorting the array could be an option, but a more efficient approach is to track the two largest numbers directly while iterating.
• We will iterate through the array once, keeping track of the two largest values, then compute their product after decrementing.
Steps:
• Initialize two variables to keep track of the largest and second-largest numbers in the array.
• Iterate through the array, updating the largest and second-largest variables as needed.
• Return the product of the decremented values of the largest and second-largest numbers.
Empty Inputs:
• The array will always contain at least two elements, so no need to handle empty inputs.
Large Inputs:
• For arrays with 500 elements, the algorithm should still run efficiently in O(n) time.
Special Values:
• If the array contains duplicate values, the two largest values might be the same. Ensure distinct indices are chosen.
Constraints:
• The solution needs to be efficient enough to handle the largest input sizes within the given constraints.
int maxProduct(vector<int>& nums) {
int max1 = INT_MIN;
int max2 = INT_MIN;
for (int num : nums) {
if (num >= max1) {
max2 = max1;
max1 = num;
} else if (num > max2) {
max2 = num;
}
}
return (max1 - 1) * (max2 - 1);
}
1 : Function Definition
int maxProduct(vector<int>& nums) {
Defines the function maxProduct, which takes a reference to a vector of integers as input and returns an integer.
2 : Variable Initialization
int max1 = INT_MIN;
Initializes max1 to the smallest possible integer value, ensuring that it will be replaced by any larger number in the array.
3 : Variable Initialization
int max2 = INT_MIN;
Initializes max2 to the smallest possible integer value, similar to max1, and will store the second largest number in the array.
4 : Loop Start
for (int num : nums) {
Starts a loop to iterate through each number in the input vector nums.
5 : Condition Check
if (num >= max1) {
Checks if the current number is greater than or equal to max1, the largest number found so far.
6 : Update Variables
max2 = max1;
Updates max2 to hold the value of max1 because max1 will be replaced by the current number.
7 : Update Variables
max1 = num;
Updates max1 to the current number because it is now the largest number found.
8 : Condition Check
} else if (num > max2) {
Checks if the current number is greater than max2, the second largest number found so far.
9 : Update Variables
max2 = num;
Updates max2 to the current number because it is the new second largest number.
10 : Return Statement
return (max1 - 1) * (max2 - 1);
Returns the product of (max1 - 1) and (max2 - 1), which is the maximum product of two distinct elements in the array, after subtracting 1 from both.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the array, because we iterate through the array once to find the two largest numbers.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) because we only need a constant amount of space to store the two largest numbers.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus