Leetcode 1433: Check If a String Can Break Another String
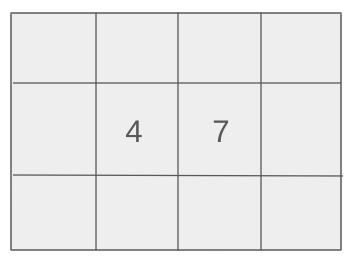
You are given two strings
s1
and s2
of the same length. You need to check if some permutation of s1
can ‘break’ some permutation of s2
, or vice-versa. A string x
can break string y
if for every character at position i
, x[i] >= y[i]
when sorted alphabetically.Problem
Approach
Steps
Complexity
Input: The input consists of two strings `s1` and `s2`, both containing only lowercase English letters.
Example: s1 = 'cat', s2 = 'abc'
Constraints:
• 1 <= s1.length == s2.length <= 10^5
• All strings consist of lowercase English letters.
Output: The output is a boolean indicating whether one permutation of `s1` can break some permutation of `s2`, or vice-versa.
Example: true
Constraints:
• The output is 'true' if one permutation can break the other, otherwise 'false'.
Goal: To determine if one permutation of `s1` can break some permutation of `s2` or vice-versa.
Steps:
• Step 1: Sort both strings `s1` and `s2`.
• Step 2: Compare the characters of the sorted strings. If at any position, a character from `s1` is less than the corresponding character from `s2`, return 'false'.
• Step 3: If no such position is found, return 'true'.
Goal: The solution should efficiently handle strings of length up to 10^5.
Steps:
• Both strings are of equal length.
• The strings consist only of lowercase English letters.
Assumptions:
• Both input strings are valid and consist of lowercase English letters.
• The strings are of equal length.
• Input: s1 = 'cat', s2 = 'abc'
• Explanation: After sorting both strings, we have 'act' and 'abc'. Since each character in 'act' is greater than or equal to the corresponding character in 'abc', 's1' can break 's2'.
• Input: s1 = 'dog', s2 = 'god'
• Explanation: After sorting both strings, we have 'dgo' and 'dgo'. Since the strings are identical, neither can break the other.
Approach: We will compare the sorted versions of both strings to determine if one string can break the other. Sorting ensures that we are comparing the lexicographically ordered characters.
Observations:
• Sorting the strings ensures that we can directly compare their characters in lexicographical order.
• By comparing sorted strings character by character, we can determine if one can break the other.
Steps:
• Step 1: Sort both `s1` and `s2`.
• Step 2: Compare each character of the sorted strings. If any character of `s1` is smaller than the corresponding character of `s2`, return false.
• Step 3: If the comparison holds for all characters, return true.
Empty Inputs:
• There are no empty inputs as the problem guarantees non-empty strings.
Large Inputs:
• Ensure the solution handles strings up to the length of 10^5 efficiently.
Special Values:
• Consider cases where the strings are identical (e.g., 'abc' and 'abc').
Constraints:
• The solution should be efficient enough to handle strings of length up to 10^5.
bool checkIfCanBreak(string s1, string s2) {
sort(s1.begin(), s1.end());
sort(s2.begin(), s2.end());
int n = s1.size();
string a = s1 < s2 ? s2: s1;
string b = s1 < s2 ? s1: s2;
// cout << a << "\n"<< b;
for(int i = 0; i < n; i++) {
if(a[i] < b[i]) return false;
}
return true;
}
1 : Function Definition
bool checkIfCanBreak(string s1, string s2) {
Defines the function `checkIfCanBreak`, which takes two strings `s1` and `s2` as input and determines whether one can break the other.
2 : Sorting
sort(s1.begin(), s1.end());
Sorts the string `s1` in lexicographical order.
3 : Sorting
sort(s2.begin(), s2.end());
Sorts the string `s2` in lexicographical order.
4 : Variable Initialization
int n = s1.size();
Stores the size of string `s1` in the variable `n`. Both strings are assumed to have the same length.
5 : String Comparison
string a = s1 < s2 ? s2: s1;
Assigns `a` to be the lexicographically larger of `s1` and `s2`.
6 : String Comparison
string b = s1 < s2 ? s1: s2;
Assigns `b` to be the lexicographically smaller of `s1` and `s2`.
7 : Loop
for(int i = 0; i < n; i++) {
Begins a loop that iterates over each character of the strings `a` and `b`.
8 : Conditional Check
if(a[i] < b[i]) return false;
Compares the characters at the same position in `a` and `b`. If any character in `a` is less than the corresponding character in `b`, returns `false`.
9 : Return Statement
return true;
If the loop completes without returning `false`, it means that every character in `a` is greater than or equal to the corresponding character in `b`, so it returns `true`.
Best Case: O(n log n) for sorting the strings.
Average Case: O(n log n) for sorting and O(n) for the comparison.
Worst Case: O(n log n) due to the sorting step.
Description: The time complexity is dominated by the sorting step.
Best Case: O(n), as we need to store the sorted versions of the strings.
Worst Case: O(n) for storing the sorted strings.
Description: The space complexity is linear, as we are storing the sorted versions of both strings.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus