Leetcode 1432: Max Difference You Can Get From Changing an Integer
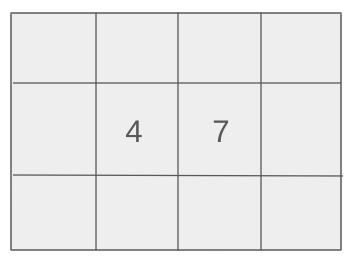
You are given an integer
num
and you must apply a set of operations twice to generate two new integers. The goal is to find the maximum difference between the results of these operations.Problem
Approach
Steps
Complexity
Input: The input consists of a single integer `num`, which represents the number you will perform operations on.
Example: num = 123
Constraints:
• 1 <= num <= 10^8
Output: The output is the maximum difference between the two integers `a` and `b` after applying the operations.
Example: 87
Constraints:
• The output is a non-negative integer representing the maximum difference.
Goal: To find the maximum difference between two new integers generated by applying two transformations to the original number.
Steps:
• Step 1: Try all combinations of `x` and `y` (0 <= x, y <= 9).
• Step 2: For each combination, replace all occurrences of `x` with `y` in `num`.
• Step 3: Calculate the resulting numbers `a` and `b`, and find the difference between them.
• Step 4: Return the maximum difference.
Goal: The solution must handle integers up to the size of 10^8 and handle operations without producing invalid outputs such as leading zeros or 0.
Steps:
• The resulting integer cannot have leading zeros.
• The resulting integer cannot be 0.
Assumptions:
• The input number `num` is guaranteed to be a valid integer in the given range.
• Input: num = 123
• Explanation: By choosing `x = 1` and `y = 9`, the first operation produces `923`. By choosing `x = 1` and `y = 0`, the second operation produces `23`. The maximum difference is `923 - 23 = 87`.
• Input: num = 408
• Explanation: By choosing `x = 4` and `y = 9`, the first operation produces `908`. By choosing `x = 4` and `y = 0`, the second operation produces `0`. The maximum difference is `908 - 0 = 408`.
Approach: We will iterate through all possible values for `x` and `y` and apply the transformations to the given number. We will then find the maximum difference between the resulting numbers.
Observations:
• The two digits `x` and `y` can be any digit from 0 to 9.
• The maximum difference will be achieved by manipulating the number to maximize one result while minimizing the other.
• By trying different combinations of `x` and `y`, we can systematically calculate all possible outcomes.
Steps:
• Step 1: Define a helper function to perform the transformation on `num`.
• Step 2: Loop through all combinations of `x` and `y` to generate potential results for both transformations.
• Step 3: Track the minimum and maximum values of `a` and `b`.
• Step 4: Return the maximum difference.
Empty Inputs:
• The problem guarantees that `num` will be at least 1, so there are no empty inputs.
Large Inputs:
• Ensure the solution can handle values up to 10^8 efficiently.
Special Values:
• Consider cases where the digits are the same (e.g., `num = 1111`) and cases with zeros (e.g., `num = 100`).
Constraints:
• Ensure that no result contains leading zeros or becomes 0.
int change(int num, int x, int y) {
int tmp = 0, ten = 1;
int digit;
while(num) {
digit = num % 10;
if(digit == x) digit = y;
tmp = ten * digit + tmp;
num /= 10;
ten *= 10;
}
return digit == 0? -1: tmp;
}
int maxDiff(int num) {
int mn = num, mx = num;
for(int i = 0; i < 10; i++)
for(int j = 0; j < 10; j++) {
if(i == j) continue;
int ret = change(num, i, j);
if(ret <= 0) continue;
mn = min(mn, ret);
mx = max(mx, ret);
}
cout << mx << " " << mn;
return mx - mn;
}
1 : Function Definition
int change(int num, int x, int y) {
Defines the `change` function that modifies the digits of a number `num`, replacing occurrences of digit `x` with digit `y`.
2 : Variable Initialization
int tmp = 0, ten = 1;
Initializes two variables: `tmp` to store the modified number, and `ten` to track the place value of the digits during the process.
3 : Variable Initialization
int digit;
Declares an integer variable `digit` to store the current digit of `num` as it is processed.
4 : Loop Constructs
while(num) {
Starts a loop that processes each digit of the number `num` until all digits are processed.
5 : Modulo Operation
digit = num % 10;
Extracts the last digit of `num` using the modulo operation.
6 : Conditional Operation
if(digit == x) digit = y;
Checks if the extracted digit is equal to `x`. If so, it replaces the digit with `y`.
7 : Digit Rebuilding
tmp = ten * digit + tmp;
Reconstructs the modified number by adding the current digit (adjusted for place value) to `tmp`.
8 : Number Update
num /= 10;
Removes the last digit of `num` by dividing it by 10.
9 : Place Value Update
ten *= 10;
Multiplies `ten` by 10 to shift the place value for the next digit.
10 : Return Statement
return digit == 0? -1: tmp;
Checks if the last processed digit is zero. If so, returns `-1` to indicate no valid modification, otherwise returns the modified number.
11 : Function Definition
int maxDiff(int num) {
Defines the `maxDiff` function that calculates the maximum difference by calling the `change` function for various digit replacements.
12 : Variable Initialization
int mn = num, mx = num;
Initializes two variables `mn` and `mx` to track the minimum and maximum numbers obtained by modifying the digits of `num`.
13 : Nested Loop
for(int i = 0; i < 10; i++)
Starts a loop that iterates through all possible digits `i` (from 0 to 9) to replace in the number `num`.
14 : Nested Loop
for(int j = 0; j < 10; j++) {
Starts an inner loop to try replacing the digit `i` with every possible digit `j` (from 0 to 9).
15 : Conditional Check
if(i == j) continue;
Checks if `i` and `j` are the same. If so, it skips this iteration as no change would occur.
16 : Function Call
int ret = change(num, i, j);
Calls the `change` function to modify `num` by replacing digit `i` with digit `j`.
17 : Condition Check
if(ret <= 0) continue;
Checks if the result of `change` is less than or equal to zero. If so, it skips the current iteration.
18 : Min/Max Update
mn = min(mn, ret);
Updates `mn` to the minimum of its current value and the result from the `change` function.
19 : Min/Max Update
mx = max(mx, ret);
Updates `mx` to the maximum of its current value and the result from the `change` function.
20 : Return Statement
return mx - mn;
Returns the difference between the maximum and minimum modified values.
Best Case: O(1), for small values of `num`.
Average Case: O(100), since we try up to 100 combinations of `x` and `y`.
Worst Case: O(100), as we are iterating over the digits 0-9 for both `x` and `y`.
Description: The time complexity is constant in terms of iterations but depends on the number of combinations for `x` and `y`.
Best Case: O(1), as no additional space is required.
Worst Case: O(1), only a few variables are needed.
Description: The space complexity is constant as we only store a few variables.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus