Leetcode 1423: Maximum Points You Can Obtain from Cards
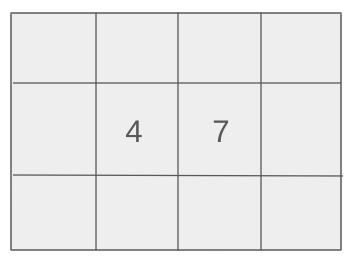
You are given an array
cardPoints
representing the points of cards arranged in a row. In each step, you can pick the first or the last card from the row. Your goal is to pick exactly k
cards and maximize the sum of the points of the cards you select.Problem
Approach
Steps
Complexity
Input: The input consists of an integer array `cardPoints` and an integer `k`, representing the cards and the number of cards to be selected respectively.
Example: [1, 2, 3, 4, 5, 6, 1], k = 3
Constraints:
• 1 <= cardPoints.length <= 10^5
• 1 <= cardPoints[i] <= 10^4
• 1 <= k <= cardPoints.length
Output: The output is an integer representing the maximum score obtained by selecting exactly `k` cards.
Example: 12
Constraints:
• The output is the maximum sum of the points of `k` cards selected from the array.
Goal: The goal is to find the maximum score by selecting exactly `k` cards from either end of the row.
Steps:
• Step 1: Use dynamic programming to track the total sum of cards taken from the left and right ends of the row.
• Step 2: Evaluate each possible way of taking cards and find the maximum sum.
• Step 3: Return the maximum score found.
Goal: The constraints ensure the input size is manageable, but the solution must still be efficient to handle up to 100,000 cards.
Steps:
• 1 <= cardPoints.length <= 10^5
• 1 <= cardPoints[i] <= 10^4
• 1 <= k <= cardPoints.length
Assumptions:
• The array `cardPoints` contains at least one element.
• You can only pick `k` cards from the beginning or the end of the row.
• Input: [1, 2, 3, 4, 5, 6, 1], k = 3
• Explanation: In this case, picking the last three cards results in the maximum sum: 1 + 6 + 5 = 12.
• Input: [9, 7, 7, 9, 7, 7, 9], k = 7
• Explanation: Here, you need to take all the cards, so the maximum sum is the sum of all the cards, which is 55.
Approach: The approach involves calculating the maximum score by considering all ways of picking `k` cards from the two ends of the row.
Observations:
• This problem involves selecting elements from the start or the end of an array.
• Dynamic programming or sliding window techniques can be applied to track the maximum score.
• We need to efficiently calculate the score for various ways of selecting `k` cards.
Steps:
• Step 1: Initialize two arrays to track the cumulative sums of cards from both ends of the array.
• Step 2: Compute the sum of cards for each possible selection of `k` cards by varying the number taken from each end.
• Step 3: Return the maximum score obtained from the possible selections.
Empty Inputs:
• There are no empty inputs since the length of the array is at least 1.
Large Inputs:
• For large inputs, ensure that the solution runs in linear time to handle up to 100,000 elements.
Special Values:
• Handle the case where all cards have the same point value, or where `k` is equal to the length of the array.
Constraints:
• The array size can be large, but the time complexity of the solution should allow for efficient computation.
int maxScore(vector<int>& card, int k) {
int n = card.size();
vector<int> frt, bck;
frt.push_back(0);
bck.push_back(0);
for(int i = 0; i < n; i++) {
frt.push_back(frt.back() + card[i]);
bck.push_back(bck.back() + card[n - 1 - i]);
}
int ans = bck[k];
for(int i = 1; i <= k; i++) {
ans = max(ans, frt[i] + bck[k - i]);
}
return ans;
}
1 : Function Definition
int maxScore(vector<int>& card, int k) {
Defines the function `maxScore` that takes a vector of integers `card` and an integer `k` as input and returns the maximum score possible.
2 : Variable Initialization
int n = card.size();
Initializes the variable `n` to the size of the `card` vector, which represents the total number of cards available.
3 : Variable Initialization
vector<int> frt, bck;
Declares two vectors `frt` and `bck`, which will store cumulative sums for the front and back sections of the `card` vector.
4 : Vector Operations
frt.push_back(0);
Pushes a `0` into the `frt` vector to represent the cumulative sum of cards from the front (starting with zero).
5 : Vector Operations
bck.push_back(0);
Pushes a `0` into the `bck` vector to represent the cumulative sum of cards from the back (starting with zero).
6 : Loop Constructs
for(int i = 0; i < n; i++) {
Begins a loop that iterates over all the cards in the `card` vector.
7 : Vector Operations
frt.push_back(frt.back() + card[i]);
For each card `i`, adds the current card's value to the cumulative sum in the `frt` vector, which tracks the sum of cards taken from the front.
8 : Vector Operations
bck.push_back(bck.back() + card[n - 1 - i]);
For each card `i`, adds the current card's value to the cumulative sum in the `bck` vector, which tracks the sum of cards taken from the back.
9 : Score Initialization
int ans = bck[k];
Initializes the variable `ans` with the sum of the first `k` cards from the back, which represents the score starting with cards from the back.
10 : Loop Constructs
for(int i = 1; i <= k; i++) {
Begins a loop that iterates through possible splits of the `k` cards between the front and the back.
11 : Score Calculation
ans = max(ans, frt[i] + bck[k - i]);
Updates the value of `ans` to be the maximum score found by splitting the `k` cards between the front and back sections.
12 : Return Statement
return ans;
Returns the maximum score found from the different possible splits of the cards.
Best Case: O(n), where n is the length of the array.
Average Case: O(n), since we process each element of the array exactly once.
Worst Case: O(n), as we need to compute the score for each possible selection of `k` cards.
Description: The time complexity is linear, making it efficient for large input sizes.
Best Case: O(n), as the space usage depends on the length of the input array.
Worst Case: O(n), since we store the cumulative sums of cards from both ends.
Description: The space complexity is linear, as we need extra space for the cumulative sums.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus