Leetcode 1422: Maximum Score After Splitting a String
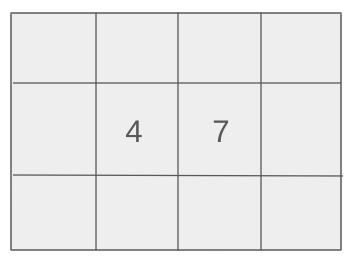
You are given a binary string
s
consisting of characters ‘0’ and ‘1’. Your task is to split the string into two non-empty substrings (a left substring and a right substring) and return the maximum score that can be achieved by splitting the string. The score is the sum of the number of ‘0’s in the left substring and the number of ‘1’s in the right substring. Find the maximum score by evaluating all possible splits.Problem
Approach
Steps
Complexity
Input: The input consists of a binary string `s` with characters '0' and '1'.
Example: "10101"
Constraints:
• 2 <= s.length <= 500
• The string `s` consists of only the characters '0' and '1'.
Output: The output is an integer representing the maximum score obtained after splitting the string `s` into two non-empty substrings.
Example: 4
Constraints:
• The score is the sum of the number of '0's in the left substring and the number of '1's in the right substring.
Goal: The goal is to find the maximum score by evaluating every possible split of the string.
Steps:
• Step 1: Traverse the string and calculate the number of '1's in the right substring.
• Step 2: For each position in the string (excluding the last), calculate the number of '0's in the left substring and the number of '1's in the right substring.
• Step 3: Track the maximum score during this traversal.
Goal: The constraints ensure the input string is of moderate size, making it feasible to evaluate all possible splits.
Steps:
• 2 <= s.length <= 500
• The string `s` contains only the characters '0' and '1'.
Assumptions:
• The string will have at least two characters.
• The string contains only '0' and '1', and we need to count occurrences of each character.
• Input: "10101"
• Explanation: In this example, the maximum score is achieved when the left substring is '1' and the right substring is '0101'. The score is calculated as 0 (zeros in the left) + 3 (ones in the right) = 3.
• Input: "1101"
• Explanation: Here, the maximum score is 3 when the left substring is '11' and the right substring is '01'. The score is calculated as 2 (zeros in the left) + 1 (ones in the right) = 3.
Approach: We can solve this by traversing the string, keeping track of the number of zeros in the left substring and the number of ones in the right substring, and updating the score at each step.
Observations:
• The number of zeros and ones in each substring must be tracked separately.
• We can traverse the string once and calculate the score for each potential split.
• We need to handle the traversal efficiently, updating counts of zeros and ones as we process the string.
Steps:
• Step 1: Initialize a counter for the number of ones in the right substring.
• Step 2: Traverse the string and for each position, update the count of zeros in the left substring and ones in the right substring.
• Step 3: Track the maximum score after each split.
Empty Inputs:
• A string of length 1 or less is not valid based on the problem constraints.
Large Inputs:
• Ensure the solution can handle strings up to length 500 efficiently.
Special Values:
• Handle cases where the string is mostly zeros or mostly ones.
Constraints:
• The string should contain at least two characters and only '0' and '1'.
int maxScore(string s) {
int rightOnes = 0, leftZeroes = 0;
for(char c: s)
if(c=='1') rightOnes++;
int score = 0;
for(int i=0; i<s.length()-1; i++){
if(s[i]=='0') leftZeroes++;
else rightOnes--;
score = max(score, leftZeroes + rightOnes);
}
return score;
}
1 : Function Definition
int maxScore(string s) {
Defines the function `maxScore` that takes a string `s` and returns the maximum score possible by splitting it into two parts.
2 : Variable Initialization
int rightOnes = 0, leftZeroes = 0;
Initializes two variables: `rightOnes` to count the number of '1's on the right part of the string, and `leftZeroes` to count the number of '0's on the left part.
3 : Loop Constructs
for(char c: s)
Begins a loop to iterate over each character `c` in the string `s`.
4 : Conditionals
if(c=='1') rightOnes++;
If the character is '1', increment the `rightOnes` counter to track the number of '1's.
5 : Variable Initialization
int score = 0;
Initializes the variable `score` to 0, which will keep track of the maximum score found during the iterations.
6 : Loop Constructs
for(int i=0; i<s.length()-1; i++){
Begins another loop to iterate through the string `s`, excluding the last character to ensure both parts of the split are non-empty.
7 : Conditionals
if(s[i]=='0') leftZeroes++;
If the character at position `i` is '0', increment the `leftZeroes` counter to track the number of '0's in the left part of the split.
8 : Conditionals
else rightOnes--;
If the character at position `i` is '1', decrement the `rightOnes` counter, reducing the number of '1's in the right part.
9 : Score Calculation
score = max(score, leftZeroes + rightOnes);
Calculates the score for the current split as the sum of `leftZeroes` and `rightOnes`, and updates the `score` to the maximum score found so far.
10 : Return Statement
return score;
Returns the maximum score found from all possible splits.
Best Case: O(n), where n is the length of the string.
Average Case: O(n), as we process each character exactly once.
Worst Case: O(n), since we need to process each character to calculate the maximum score.
Description: The time complexity is linear, as we only traverse the string once.
Best Case: O(1), since the space usage does not depend on the input size.
Worst Case: O(1), as the solution uses only a few counters to keep track of zeros and ones.
Description: The space complexity is constant since we only store a few counters.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus