Leetcode 1415: The k-th Lexicographical String of All Happy Strings of Length n
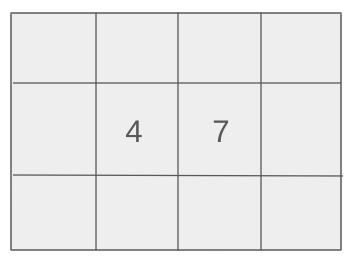
A happy string consists of only the letters ‘a’, ‘b’, and ‘c’, and no two consecutive characters in the string are the same. Given two integers n and k, return the kth happy string of length n sorted in lexicographical order, or an empty string if there are fewer than k happy strings of length n.
Problem
Approach
Steps
Complexity
Input: The input consists of two integers n and k.
Example: n = 2, k = 4
Constraints:
• 1 <= n <= 10
• 1 <= k <= 100
Output: Return the kth happy string of length n or an empty string if there are fewer than k happy strings of length n.
Example: For n = 2, k = 4, the output is 'ba'.
Constraints:
Goal: Generate all happy strings of length n in lexicographical order and return the kth string.
Steps:
• Use backtracking to generate all possible happy strings.
• Sort the strings lexicographically.
• Return the kth string, or an empty string if there are fewer than k happy strings.
Goal: Ensure that the values of n and k are within the given bounds.
Steps:
• 1 <= n <= 10
• 1 <= k <= 100
Assumptions:
• The value of k will always be within the range for the given n.
• Input: Input: n = 2, k = 4
• Explanation: The list of happy strings of length 2 is ['ab', 'ac', 'ba', 'bc', 'ca', 'cb']. The 4th string is 'ba'.
• Input: Input: n = 3, k = 6
• Explanation: The list of happy strings of length 3 is ['aba', 'abc', 'aca', 'acb', 'bab', 'bac', 'bca', 'bcb', 'cab', 'cac', 'cba', 'cbc']. The 6th string is 'bca'.
Approach: Generate all happy strings of length n using a backtracking approach and return the kth string.
Observations:
• The backtracking approach can be used to construct the strings while ensuring the condition of no two consecutive characters being the same.
• Backtracking allows us to efficiently explore all possible combinations of characters while checking for the happy string conditions.
Steps:
• Initialize an empty string.
• Recursively add characters from the set ['a', 'b', 'c'] to the string, ensuring no two consecutive characters are the same.
• Sort the strings lexicographically and return the kth string.
Empty Inputs:
• k is larger than the number of possible happy strings of length n.
Large Inputs:
• For n = 10, handle cases where the number of strings is large.
Special Values:
• If k exceeds the number of possible happy strings of length n, return an empty string.
Constraints:
• Ensure the function works efficiently within the given bounds for both n and k.
string ans = "";
int cnt = 0;
int k, n;
string getHappyString(int n, int k) {
this->k = k;
this->n = n;
string tmp = "";
bt(-1, tmp);
return ans;
}
void bt(int prv, string &tmp) {
if(tmp.size() == n) {
cnt++;
if(cnt == k){
ans = tmp;
}
return;
}
for(int i = 0; i < 3; i++) {
if(prv == i) continue;
if(i == 0) {
tmp += 'a';
} else if(i == 1) {
tmp += 'b';
} else if(i == 2) {
tmp += 'c';
}
bt(i, tmp);
tmp.pop_back();
}
}
1 : Variable Initialization
string ans = "";
Initializes an empty string `ans` to store the final happy string when found.
2 : Variable Initialization
int cnt = 0;
Initializes a counter `cnt` to keep track of how many happy strings have been generated.
3 : Variable Declaration
int k, n;
Declares two integer variables `k` (the index of the desired happy string) and `n` (the length of the happy string).
4 : Function Definition
string getHappyString(int n, int k) {
Defines the function `getHappyString`, which takes two integers `n` (length of the string) and `k` (the desired string's lexicographical index).
5 : Assignment
this->k = k;
Assigns the value of `k` to the member variable `k` to be used by the recursive function.
6 : Assignment
this->n = n;
Assigns the value of `n` to the member variable `n` for later use in the recursive function.
7 : Variable Initialization
string tmp = "";
Initializes an empty string `tmp` that will be used to build potential happy strings.
8 : Function Call
bt(-1, tmp);
Calls the recursive function `bt` with the initial previous character index `-1` and the empty string `tmp`.
9 : Return Statement
return ans;
Returns the happy string `ans` once the `k`-th string has been found by the recursive function.
10 : Function Definition
void bt(int prv, string &tmp) {
Defines the recursive helper function `bt`, which generates all possible happy strings and checks if the `k`-th string is reached.
11 : Base Case
if(tmp.size() == n) {
Checks if the current string `tmp` has reached the desired length `n`. If so, it proceeds to count it.
12 : Increment Operation
cnt++;
Increments the counter `cnt` each time a string of length `n` is generated.
13 : Conditional Statement
if(cnt == k){
Checks if the counter `cnt` has reached the desired `k`-th happy string. If so, it stores the string in `ans`.
14 : Assignment
ans = tmp;
Assigns the current string `tmp` to the variable `ans` when the `k`-th happy string is found.
15 : Return Statement
return;
Returns from the recursive function once the desired string has been found or the base case is reached.
16 : Loop Constructs
for(int i = 0; i < 3; i++) {
Begins a for loop that iterates over the three possible characters ('a', 'b', 'c') to add to the string `tmp`.
17 : Control Flow
if(prv == i) continue;
Skips the current iteration if the character `i` is the same as the previous character (`prv`), ensuring no two adjacent characters are the same.
18 : String Manipulation
if(i == 0) {
Checks if the current iteration is for the character 'a'.
19 : String Manipulation
tmp += 'a';
Adds the character 'a' to the string `tmp`.
20 : Conditional Statement
} else if(i == 1) {
Checks if the current iteration is for the character 'b'.
21 : String Manipulation
tmp += 'b';
Adds the character 'b' to the string `tmp`.
22 : Conditional Statement
} else if(i == 2) {
Checks if the current iteration is for the character 'c'.
23 : String Manipulation
tmp += 'c';
Adds the character 'c' to the string `tmp`.
24 : Function Call
bt(i, tmp);
Recursively calls the function `bt` with the new character added to `tmp` and the current character `i` as `prv`.
25 : String Manipulation
tmp.pop_back();
Removes the last character added to `tmp` in preparation for the next iteration of the loop.
Best Case: O(1), if k is very small.
Average Case: O(3^n), as we need to consider all possible combinations of characters.
Worst Case: O(3^n), since we may have to generate all possible strings.
Description: The time complexity grows exponentially with n due to the 3 choices at each step.
Best Case: O(1), if we are only generating a few strings.
Worst Case: O(3^n), to store the strings generated.
Description: The space complexity is proportional to the number of strings generated.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus