Leetcode 1414: Find the Minimum Number of Fibonacci Numbers Whose Sum Is K
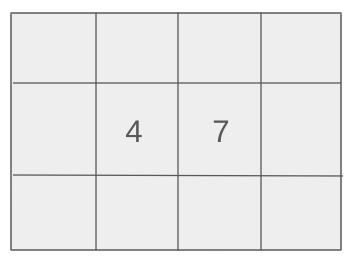
You are given an integer k, and you need to determine the minimum number of Fibonacci numbers whose sum equals k. Note that the same Fibonacci number can be used multiple times. The Fibonacci sequence is defined as F1 = 1, F2 = 1, and Fn = Fn-1 + Fn-2 for n > 2.
Problem
Approach
Steps
Complexity
Input: The input consists of a single integer k.
Example: k = 8
Constraints:
• 1 <= k <= 10^9
Output: Return the minimum number of Fibonacci numbers whose sum is equal to k.
Example: For k = 8, output 1 as only one Fibonacci number is needed.
Constraints:
Goal: Determine the minimum number of Fibonacci numbers that sum to k.
Steps:
• Generate all Fibonacci numbers less than or equal to k.
• Greedily subtract the largest Fibonacci numbers from k until k becomes 0.
• Count the number of Fibonacci numbers used.
Goal: Ensure that the number k falls within the specified range.
Steps:
• 1 <= k <= 10^9
Assumptions:
• The given number k is always solvable with Fibonacci numbers.
• Input: Input: k = 8
• Explanation: The Fibonacci sequence is [1, 1, 2, 3, 5, 8]. For k = 8, we can directly use the Fibonacci number 8, so the answer is 1.
Approach: To solve this problem, generate all Fibonacci numbers less than or equal to k and greedily select the largest Fibonacci numbers that sum to k.
Observations:
• The Fibonacci numbers grow exponentially, so we can limit the number of Fibonacci numbers we need to check.
• Greedy selection from the largest Fibonacci numbers should work well here.
• We can generate Fibonacci numbers up to k, then iteratively subtract the largest Fibonacci numbers from k until we reach 0.
Steps:
• Generate Fibonacci numbers up to k.
• Iterate through the Fibonacci numbers starting from the largest and subtract from k.
• Keep track of how many Fibonacci numbers are used.
Empty Inputs:
• There are no empty inputs since k is always a positive integer.
Large Inputs:
• For large values of k near 10^9, ensure the Fibonacci sequence is generated efficiently.
Special Values:
• When k is a Fibonacci number itself, the solution should return 1.
Constraints:
• Ensure the correct implementation for all valid inputs within the given constraints.
int findMinFibonacciNumbers(int k) {
vector<int> arr = {1, 1};
while(arr[arr.size() - 1] + arr[arr.size() - 2] <= k) {
arr.push_back(arr[arr.size() - 1] + arr[arr.size() - 2]);
}
set<int> cnt;
int i = arr.size() -1;
while(k > 0) {
while(i >= 0 && arr[i] > k) i--;
if(i == -1) break;
k -= arr[i];
cnt.insert(arr[i]);
}
return cnt.size();
}
1 : Function Definition
int findMinFibonacciNumbers(int k) {
This line defines the function `findMinFibonacciNumbers`, which takes an integer `k` as input and returns an integer representing the minimum number of Fibonacci numbers whose sum equals `k`.
2 : Variable Initialization
vector<int> arr = {1, 1};
Initializes a vector `arr` containing the first two Fibonacci numbers, `1` and `1`.
3 : Loop Constructs
while(arr[arr.size() - 1] + arr[arr.size() - 2] <= k) {
This while loop continues to generate Fibonacci numbers and adds them to `arr` as long as the sum of the last two Fibonacci numbers is less than or equal to `k`.
4 : Vector Operations
arr.push_back(arr[arr.size() - 1] + arr[arr.size() - 2]);
Adds the next Fibonacci number to the vector `arr` by summing the last two numbers in the vector.
5 : Set Operations
set<int> cnt;
Initializes a set `cnt` to store unique Fibonacci numbers that contribute to the sum `k`.
6 : Variable Initialization
int i = arr.size() -1;
Initializes the variable `i` to the index of the last element in the `arr` vector, representing the largest Fibonacci number.
7 : Loop Constructs
while(k > 0) {
Begins a while loop that runs until `k` becomes 0, indicating that the sum of selected Fibonacci numbers has been achieved.
8 : Loop Constructs
while(i >= 0 && arr[i] > k) i--;
In this inner while loop, the index `i` is decremented until a Fibonacci number less than or equal to `k` is found.
9 : Control Flow
if(i == -1) break;
If no suitable Fibonacci number is found (i.e., `i` becomes -1), the loop is exited.
10 : Arithmetic Operations
k -= arr[i];
Decreases `k` by the current Fibonacci number `arr[i]`.
11 : Set Operations
cnt.insert(arr[i]);
Inserts the selected Fibonacci number `arr[i]` into the set `cnt` to ensure each number is counted only once.
12 : Return Statement
return cnt.size();
Returns the size of the set `cnt`, which represents the minimum number of Fibonacci numbers required to sum up to `k`.
Best Case: O(log k), due to the exponential growth of Fibonacci numbers.
Average Case: O(log k), since we need to process Fibonacci numbers up to k.
Worst Case: O(log k), as the number of Fibonacci numbers required is small relative to k.
Description: The time complexity is logarithmic relative to k due to the fast growth of the Fibonacci sequence.
Best Case: O(log k), as the space for the Fibonacci numbers is proportional to the logarithm of k.
Worst Case: O(log k), for storing the Fibonacci numbers up to k.
Description: The space complexity is also logarithmic because we only need to store a small number of Fibonacci numbers.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus