Leetcode 1404: Number of Steps to Reduce a Number in Binary Representation to One
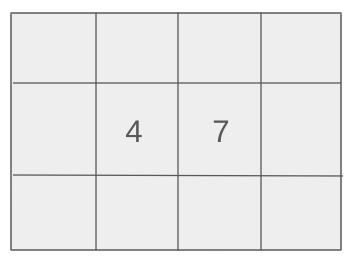
You are given a binary string representing a non-negative integer. The task is to determine the number of steps required to reduce this number to 1, following the rules: divide by 2 if the number is even, or add 1 if it is odd. Return the total number of steps required.
Problem
Approach
Steps
Complexity
Input: The input is a binary string representing a non-negative integer.
Example: "1111"
Constraints:
• 1 <= s.length <= 500
• s consists of characters '0' or '1'
• s[0] == '1'
Output: The output is an integer representing the number of steps required to reduce the given number to 1.
Example: 7
Constraints:
• The output should be the total number of steps to reduce the number to 1.
Goal: The goal is to count how many operations are needed to reduce the number to 1.
Steps:
• Convert the binary string to a decimal integer.
• For each step, check if the number is odd or even.
• If the number is odd, increment it by 1. If it's even, divide it by 2.
• Keep track of the number of operations until the number becomes 1.
Goal: The input binary string should be between 1 and 500 characters long, and the string must represent a non-negative integer.
Steps:
• The string contains only '0's and '1's.
• The first character of the string is '1'.
Assumptions:
• The input string is valid and represents a non-negative integer.
• The problem will always have a solution as the number will eventually reduce to 1.
• Input: Input: "1111"
• Explanation: The binary representation '1111' corresponds to the number 15. It takes 7 steps to reduce 15 to 1 by following the rules of even and odd operations.
• Input: Input: "100"
• Explanation: The binary representation '100' corresponds to the number 4. It takes 3 steps to reduce 4 to 1.
Approach: The approach is to repeatedly perform the appropriate operation (add 1 or divide by 2) until the number reaches 1, while counting the steps.
Observations:
• We can represent the number as a binary string, which makes it easy to check if the number is odd or even.
• We will simulate the process of reducing the number by performing the required operations, counting each step.
Steps:
• 1. Convert the binary string to a decimal integer.
• 2. While the number is greater than 1, check if it is even or odd.
• 3. If the number is even, divide it by 2. If it is odd, add 1.
• 4. Count the steps and continue until the number becomes 1.
Empty Inputs:
• The string will always have at least one character, as it represents a valid binary number.
Large Inputs:
• Ensure the solution works for binary strings up to the maximum length of 500 characters.
Special Values:
• What if the binary string is already 1? This case should immediately return 0.
Constraints:
• The solution should be efficient enough to handle up to 500-character long binary strings.
int numSteps(string s) {
int idx = s.size() - 1, cnt = 0;
while(idx > 0) {
if(s[idx] == '0') {
cnt++;
idx--;
} else {
int tmp = idx;
while(idx >= 0 && s[idx] == '1') idx--;
cnt++; // add one
if(idx >= 0) {
s[idx] = '1';
cnt += (tmp-idx); // divide by 2
} else {
cnt += (tmp-idx);
}
}
}
return cnt;
}
1 : Method Definition
int numSteps(string s) {
Defines the method `numSteps`, which takes a binary string `s` and calculates the number of steps to transform it into a string of all '0's.
2 : Variable Initialization
int idx = s.size() - 1, cnt = 0;
Initializes two variables: `idx` to point to the last character of the string and `cnt` to track the number of steps taken.
3 : Loop Start
while(idx > 0) {
Starts a `while` loop to iterate through the string from the end towards the beginning, as long as `idx` is greater than 0.
4 : Condition Check
if(s[idx] == '0') {
Checks if the current character is '0'. If so, it increments the step count and moves to the previous character.
5 : Count Increment
cnt++;
Increments the `cnt` variable by 1 when encountering a '0' at the current index.
6 : Index Decrement
idx--;
Decrements the `idx` variable to move to the previous character in the string.
7 : Else Block Start
} else {
Starts the `else` block for handling the case when the current character is '1'.
8 : Temporary Index Save
int tmp = idx;
Saves the current index (`idx`) to `tmp` before processing the '1' character.
9 : Inner Loop Start
while(idx >= 0 && s[idx] == '1') idx--;
Moves the `idx` pointer leftward while encountering '1' characters, skipping over consecutive '1's.
10 : Step Increment
cnt++; // add one
Increments `cnt` by 1 after encountering a '1' and moving past any consecutive '1's.
11 : Check for Index Validity
if(idx >= 0) {
Checks if there are still remaining characters in the string to process.
12 : Toggle Character
s[idx] = '1';
Sets the character at the current index to '1', simulating the operation of transforming a '1' into a '0'.
13 : Step Adjustment
cnt += (tmp-idx); // divide by 2
Adds the difference between the saved index (`tmp`) and the current index (`idx`) to `cnt`, simulating a 'divide by 2' operation.
14 : Else Block End
} else {
Handles the case where there are no more characters to process in the string.
15 : Final Adjustment
cnt += (tmp-idx);
Adds the difference between `tmp` and `idx` to `cnt` when the loop ends, finalizing the step count.
16 : Return Result
return cnt;
Returns the total number of steps (`cnt`) needed to transform the input binary string into a string of all '0's.
Best Case: O(1), when the input is already '1'.
Average Case: O(n), where n is the length of the binary string.
Worst Case: O(n), as we need to check each bit of the binary string and perform operations.
Description:
Best Case: O(1), as no extra space is required beyond basic variables.
Worst Case: O(1), since we only need a few variables for the calculation.
Description:
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus