Leetcode 1387: Sort Integers by The Power Value
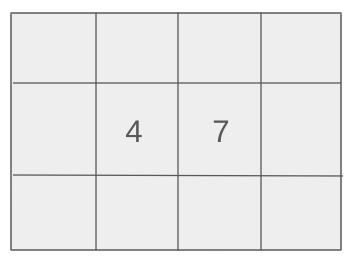
The task is to find the kth integer in the range [lo, hi] sorted by the number of steps required to reach 1 using the Collatz conjecture rules. The power of a number is the number of steps needed to reach 1, following the rules: if the number is even, divide it by 2, and if it’s odd, multiply it by 3 and add 1.
Problem
Approach
Steps
Complexity
Input: You are given three integers lo, hi, and k. The integers lo and hi represent the range of numbers to check, and k is the position of the number to return after sorting by power value.
Example: For lo = 10, hi = 15, and k = 2, the second number in the sorted list of powers is 11.
Constraints:
• 1 <= lo <= hi <= 1000
• 1 <= k <= hi - lo + 1
Output: Return the kth integer from the sorted list of integers based on their power values.
Example: For lo = 10, hi = 15, and k = 2, the output is 11.
Constraints:
• The result will always be a valid integer within the specified range.
Goal: The goal is to calculate the number of steps (power) for each integer in the range and return the kth integer after sorting them by their power values.
Steps:
• 1. Compute the power of each number in the range [lo, hi].
• 2. Sort the numbers based on their power values. If two numbers have the same power, sort them by their value.
• 3. Return the kth number from the sorted list.
Goal: The function must efficiently handle ranges within the specified constraints.
Steps:
• The range [lo, hi] can have at most 1000 elements.
• k is guaranteed to be within the range of numbers [lo, hi].
Assumptions:
• All integers in the range [lo, hi] will eventually transform into 1.
• The power values of all integers will fit within a 32-bit signed integer.
• Input: For lo = 5, hi = 9, k = 3, the sorted list of powers is [8, 6, 5, 7, 9], so the third number is 5.
• Explanation: The power of each integer in the range [5, 9] is calculated. The numbers are then sorted by their power values, and the kth number is returned.
Approach: To solve this problem, we will compute the power for each integer in the range [lo, hi], sort them by their power values, and return the kth element.
Observations:
• We need to calculate the Collatz steps for each number efficiently.
• Memoization can be used to store intermediate results to avoid redundant calculations.
• A recursive approach with memoization can be applied to compute the power values for each integer.
Steps:
• 1. Define a recursive function to calculate the power of a number using the Collatz conjecture.
• 2. Memoize the results to optimize repeated calculations.
• 3. Create a list of tuples containing the power value and the integer.
• 4. Sort the list based on power values, and if necessary, by integer value.
• 5. Return the kth element from the sorted list.
Empty Inputs:
• The input range [lo, hi] will always have at least one integer.
Large Inputs:
• The function needs to efficiently compute the power for numbers in the range up to 1000.
Special Values:
• Edge cases where lo and hi are the same value should be handled properly.
Constraints:
• The function must work efficiently within the provided input constraints.
map<int, int> memo;
int dig(int num) {
if(num == 1) return 0;
if(memo.count(num)) return memo[num];
if(num % 2 == 0)
return memo[num] = dig(num / 2) + 1;
return memo[num] = dig(3 * num + 1) + 1;
}
int getKth(int lo, int hi, int k) {
vector<pair<int,int>> ans;
for(int i = lo; i <= hi; i++) {
int tmp = dig(i);
ans.push_back({tmp, i});
}
sort(ans.begin(), ans.end());
return ans[k - 1].second;
}
1 : Data Structures
map<int, int> memo;
Declare a memoization map `memo` to store previously computed results for the 'dig' function, optimizing repeated calculations.
2 : Function Definition
int dig(int num) {
Define the recursive function `dig` that calculates the number of steps to reduce a number to 1 using the 3x + 1 problem's rules.
3 : Base Case
if(num == 1) return 0;
If the number is 1, return 0 since no steps are needed.
4 : Memoization
if(memo.count(num)) return memo[num];
Check if the result for the current number has been computed before and return it from the memoization map.
5 : Condition
if(num % 2 == 0)
If the number is even, apply the rule `num / 2`.
6 : Recursion
return memo[num] = dig(num / 2) + 1;
Recursively call `dig(num / 2)` and store the result in `memo[num]`, adding 1 to account for the step.
7 : Recursion
return memo[num] = dig(3 * num + 1) + 1;
If the number is odd, apply the rule `3 * num + 1`, recursively call `dig(3 * num + 1)`, and store the result in `memo[num]`.
8 : Function Definition
int getKth(int lo, int hi, int k) {
Define the function `getKth` to find the kth number in the range [lo, hi] based on the number of steps from `dig`.
9 : Data Structures
vector<pair<int,int>> ans;
Declare a vector `ans` of pairs to store the computed step counts and the corresponding numbers.
10 : Loop
for(int i = lo; i <= hi; i++) {
Loop through all numbers in the range [lo, hi].
11 : Function Call
int tmp = dig(i);
Call the `dig` function to get the number of steps required to reduce the current number `i` to 1.
12 : Data Structures
ans.push_back({tmp, i});
Store the result (step count `tmp` and number `i`) as a pair in the vector `ans`.
13 : Sorting
sort(ans.begin(), ans.end());
Sort the vector `ans` based on the step count in ascending order, ensuring that the number with the fewest steps comes first.
14 : Return Statement
return ans[k - 1].second;
Return the second element (the number) of the kth pair in the sorted vector `ans`.
Best Case: O(n log n), where n is the number of integers in the range [lo, hi].
Average Case: O(n log n), considering sorting of the numbers based on power values.
Worst Case: O(n log n), where n is the number of integers in the range and log n is the complexity of sorting.
Description: The primary time complexity is due to sorting the numbers by their power values.
Best Case: O(n), for storing the memoized results and the list of integers.
Worst Case: O(n), where n is the number of integers in the range [lo, hi], for storing the power values and the sorted list.
Description: The space complexity is determined by the storage of the memoized results and the list of integers.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus