Leetcode 1347: Minimum Number of Steps to Make Two Strings Anagram
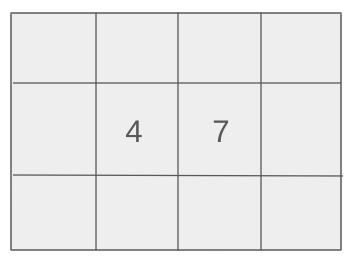
You are given two strings s and t of the same length. In one step, you can choose any character of t and replace it with another character. Return the minimum number of steps to make t an anagram of s.
Problem
Approach
Steps
Complexity
Input: The input consists of two strings, s and t, each containing lowercase English letters.
Example: For s = 'abc' and t = 'cba', no steps are required as the strings are already anagrams.
Constraints:
• 1 <= s.length <= 5 * 10^4
• s.length == t.length
• s and t consist of lowercase English letters only.
Output: Return the minimum number of operations required to convert string t into an anagram of string s.
Example: For s = 'abcdef' and t = 'abcfgh', the output is 2 as 'g' and 'h' must be replaced.
Constraints:
• The result should be within 10^-5 of the correct value.
Goal: The goal is to determine the number of replacements required to make t an anagram of s.
Steps:
• 1. Count the frequency of each character in both strings s and t.
• 2. Calculate the difference in frequency for each character between s and t.
• 3. Sum the positive differences to get the minimum number of changes required.
Goal: Handle all valid inputs efficiently within the problem constraints.
Steps:
• Both strings have the same length.
• Both strings consist of lowercase English letters only.
Assumptions:
• The strings s and t are always non-empty and have the same length.
• Input: Example 1: s = 'abc', t = 'cba'
• Explanation: Both strings are already anagrams of each other, so no operations are required.
• Input: Example 2: s = 'abcdef', t = 'abcfgh'
• Explanation: Two characters ('g' and 'h') need to be replaced to make t an anagram of s.
• Input: Example 3: s = 'hello', t = 'world'
• Explanation: Four characters ('w', 'r', 'o', 'd') need to be replaced to make t an anagram of s.
Approach: The approach is to compare the frequency of characters in both strings and count how many characters in t need to be replaced to match s.
Observations:
• Both strings are of the same length, and we only need to adjust the frequency of characters.
• We can use a frequency counter to track how many characters are overrepresented or underrepresented in string t.
Steps:
• 1. Initialize a count array for both strings to track the frequency of each character.
• 2. For each character in s and t, update the respective count arrays.
• 3. Calculate the total number of replacements needed by summing the positive differences between the count arrays.
Empty Inputs:
• There are no empty inputs, as both strings have a length of at least 1.
Large Inputs:
• The solution must efficiently handle large strings with up to 50,000 characters.
Special Values:
• If the strings are already anagrams, no steps are required.
Constraints:
• Ensure to handle cases where there are multiple instances of the same character in both strings.
int minSteps(string s, string t) {
vector<int> count(26, 0);
for(int i = 0; i < s.length(); i++) {
count[s[i] - 'a']++;
count[t[i] - 'a']--;
}
int step = 0;
for(int num : count)
if(num > 0) step += num;
return step;
}
1 : Function Declaration
int minSteps(string s, string t) {
Declares a function to calculate the minimum steps to make string `s` an anagram of string `t`.
2 : Initialization
vector<int> count(26, 0);
Initializes a frequency array `count` to track the differences in character occurrences between `s` and `t`.
3 : Loop to Count Frequency
for(int i = 0; i < s.length(); i++) {
Iterates through each character in `s` and `t` to calculate frequency differences.
4 : Increment Frequency
count[s[i] - 'a']++;
Increments the frequency count for the character in `s`.
5 : Decrement Frequency
count[t[i] - 'a']--;
Decrements the frequency count for the character in `t`.
6 : Variable Declaration
int step = 0;
Declares a variable `step` to store the total number of steps required.
7 : Iterate Frequency Array
for(int num : count)
Iterates through the frequency array to calculate the positive mismatches.
8 : Count Mismatches
if(num > 0) step += num;
Adds the positive mismatches to `step`, representing characters that need to be adjusted.
9 : Return Result
return step;
Returns the total number of steps required to make the strings anagrams.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is linear, O(n), where n is the length of the strings.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant, O(1), since the space used for character counts is fixed.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus