Leetcode 1328: Break a Palindrome
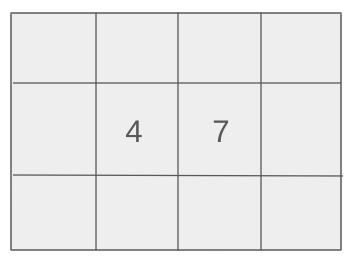
Given a string
palindrome
that is a palindrome, you need to replace exactly one character with any lowercase English letter such that the resulting string is no longer a palindrome. The resulting string should be the lexicographically smallest string possible. If it is not possible to change the string to make it non-palindromic, return an empty string.Problem
Approach
Steps
Complexity
Input: The input consists of a string `palindrome` which is guaranteed to be a palindrome and consists of only lowercase English letters.
Example: For palindrome = 'abba', the string is a palindrome and needs to be modified to be non-palindromic.
Constraints:
• 1 <= palindrome.length <= 1000
• palindrome consists of only lowercase English letters.
Output: The output should be the modified string that is no longer a palindrome and is the lexicographically smallest possible result. If no such modification is possible, return an empty string.
Example: For palindrome = 'abba', the output could be 'aaba'.
Constraints:
Goal: To break the palindrome by replacing one character to make the string non-palindromic, while ensuring the result is the lexicographically smallest possible.
Steps:
• 1. Traverse the string from left to right up to the midpoint.
• 2. If a character is not 'a', replace it with 'a' and return the modified string.
• 3. If no modification is made, change the last character to 'b' if possible.
• 4. If the length of the string is 1, return an empty string as no modification is possible.
Goal: The string must be a palindrome and adhere to the given length and character constraints.
Steps:
• 1 <= palindrome.length <= 1000
• palindrome consists of only lowercase English letters.
Assumptions:
• The input string is guaranteed to be a palindrome.
• Input: For palindrome = 'abccba', the output is 'aaccba'.
• Explanation: By changing the first 'b' to 'a', the string is no longer a palindrome and the result is lexicographically the smallest.
• Input: For palindrome = 'a', the output is an empty string.
• Explanation: Since a single character string cannot be modified to become non-palindromic, the function returns an empty string.
Approach: The solution uses a greedy approach to replace characters in the palindrome. The algorithm first checks if a character can be replaced with 'a' to minimize the string lexicographically. If no replacement is possible, the last character is changed to 'b' to break the palindrome.
Observations:
• The palindrome property can be exploited by checking each character from left to right.
• We need to consider edge cases where the string is too short or already in the form where no modification is possible.
Steps:
• 1. Iterate through the string from left to right up to the middle.
• 2. For each character, check if it's not 'a'. If so, replace it with 'a' and return the result.
• 3. If no change is made by the end of the loop, modify the last character to 'b'.
• 4. If the string has only one character, return an empty string since it cannot be modified.
Empty Inputs:
• An empty string will not be provided as input, based on the problem constraints.
Large Inputs:
• The algorithm should efficiently handle strings with up to 1000 characters.
Special Values:
• If the string is of length 1, return an empty string.
Constraints:
• The solution must handle strings that are palindromes and only contain lowercase English letters.
string breakPalindrome(string palindrome) {
int n = palindrome.size();
for(int i = 0; i < n / 2; i++) {
if(palindrome[i] != 'a') {
palindrome[i] = 'a';
return palindrome;
}
}
palindrome[n - 1] = 'b';
return n < 2 ? "" : palindrome;
}
1 : Function Declaration
string breakPalindrome(string palindrome) {
The function 'breakPalindrome' is declared. It takes a string 'palindrome' as input and returns a string as the output after breaking the palindrome.
2 : String Length Calculation
int n = palindrome.size();
The size of the input string 'palindrome' is calculated and stored in variable 'n'. This is used to determine the length of the string and guide the loop for processing.
3 : Loop Setup
for(int i = 0; i < n / 2; i++) {
A loop is initiated to iterate over the first half of the string. The loop ensures that the palindrome is checked from the left side, as the second half is symmetric.
4 : Check for Non-'a' Character
if(palindrome[i] != 'a') {
Inside the loop, the function checks if the current character is not 'a'. If it is not 'a', the character will be changed to 'a' to make the string lexicographically smaller.
5 : Character Replacement
palindrome[i] = 'a';
If the current character is not 'a', it is replaced with 'a', and the function immediately returns the modified string, effectively breaking the palindrome.
6 : Return Modified String
return palindrome;
The function returns the modified string after making the change to break the palindrome.
7 : Replace Last Character
palindrome[n - 1] = 'b';
If no non-'a' character was found, the function replaces the last character of the palindrome with 'b' to break the palindrome.
8 : Return Result
return n < 2 ? "" : palindrome;
If the string length is less than 2, it returns an empty string (as it’s impossible to break a single character palindrome). Otherwise, it returns the modified palindrome.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: In all cases, the algorithm iterates through the string once, which results in a time complexity of O(n), where n is the length of the string.
Best Case: O(1)
Worst Case: O(n)
Description: The space complexity is O(1) for the in-place modification of the string, but if a new string is created, it would require O(n) space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus