Leetcode 1310: XOR Queries of a Subarray
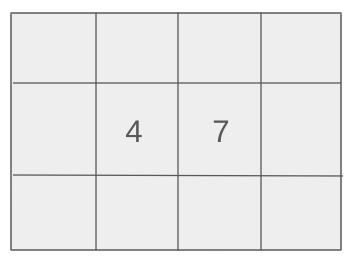
You are given an array of positive integers
arr
and an array of queries. For each query [lefti, righti]
, calculate the XOR of elements from index lefti
to righti
(inclusive). Return an array answer
where each element corresponds to the XOR result of the respective query.Problem
Approach
Steps
Complexity
Input: The input consists of an array of positive integers and an array of queries, where each query contains two integers representing a range in the array.
Example: Input: arr = [2, 5, 7, 10], queries = [[0,2],[1,3],[2,3],[0,1]]
Constraints:
• 1 <= arr.length, queries.length <= 3 * 10^4
• 1 <= arr[i] <= 10^9
• queries[i].length == 2
• 0 <= lefti <= righti < arr.length
Output: The output is an array of integers where each element is the XOR result of the corresponding query.
Example: Output: [4, 5, 13, 7]
Constraints:
• Each element of the output corresponds to the XOR result for the respective query.
Goal: Calculate the XOR of elements between indices `lefti` and `righti` for each query efficiently.
Steps:
• Use prefix XOR array to precompute XOR values for the array.
• For each query, calculate the XOR from the prefix array using the formula: `prefix[righti + 1] ^ prefix[lefti]`.
Goal: The solution should handle up to 30,000 queries and array elements efficiently.
Steps:
• Ensure that the algorithm runs in optimal time for the input size constraints.
Assumptions:
• The array and queries are well-formed and follow the given constraints.
• Input: Input: arr = [2, 5, 7, 10], queries = [[0,2],[1,3],[2,3],[0,1]]
• Explanation: The XOR values for queries are computed by applying the XOR operation on the array elements between indices `lefti` and `righti`.
Approach: We can solve the problem efficiently by using a prefix XOR array, which allows us to compute the XOR for any range in constant time.
Observations:
• The XOR of a range of elements can be derived from a prefix XOR array.
• Precompute the XOR for all elements up to index `i` in a prefix array. Then, for each query, the result can be derived in constant time.
Steps:
• Initialize a prefix XOR array where `prefix[i]` stores the XOR of elements from index 0 to `i-1`.
• For each query, use the formula `prefix[righti + 1] ^ prefix[lefti]` to compute the XOR for the range.
Empty Inputs:
• If the array is empty, there are no queries to process.
Large Inputs:
• For large arrays and queries, ensure that the solution handles up to 30,000 elements efficiently.
Special Values:
• If the elements in the array are all the same, the XOR result for any range may be predictable.
Constraints:
• The solution must handle arrays with up to 30,000 elements and queries efficiently.
vector<int> xorQueries(vector<int>& arr, vector<vector<int>>& q) {
int n = arr.size();
vector<int> xr(n +1,0);
for(int i = 1; i < n +1; i++)
xr[i] = xr[i - 1] ^ arr[i - 1];
vector<int> res;
for(int i = 0; i < q.size(); i++) {
auto &v = q[i];
res.push_back(xr[v[1]+1] ^ xr[v[0]]);
}
return res;
}
1 : Function Declaration
vector<int> xorQueries(vector<int>& arr, vector<vector<int>>& q) {
The function 'xorQueries' is defined with two parameters: a vector 'arr' which contains the array elements and a 2D vector 'q' which holds the queries. Each query specifies a range for which the XOR is computed.
2 : Variable Initialization
int n = arr.size();
The variable 'n' is initialized to the size of the input array 'arr'. This value is used to manage array bounds during the processing of queries.
3 : Vector Initialization
vector<int> xr(n +1,0);
A vector 'xr' is initialized with size 'n+1' to store the cumulative XOR values. The extra space is to handle the prefix sum logic efficiently.
4 : Loop Control
for(int i = 1; i < n +1; i++)
This for loop starts from index 1 and iterates through the array to compute the cumulative XOR for each element.
5 : XOR Operation
xr[i] = xr[i - 1] ^ arr[i - 1];
In each iteration, the cumulative XOR at index 'i' is calculated by XORing the previous cumulative value with the current element of the array. This builds a prefix XOR array.
6 : Vector Initialization
vector<int> res;
An empty vector 'res' is initialized to store the results of each query.
7 : Loop Control
for(int i = 0; i < q.size(); i++) {
This loop iterates through each query in the 2D vector 'q'. Each query specifies a range of indices for which the XOR operation needs to be performed.
8 : Query Processing
auto &v = q[i];
The current query is extracted by referencing the query vector 'v', which contains two indices representing the range for XOR computation.
9 : XOR Operation
res.push_back(xr[v[1]+1] ^ xr[v[0]]);
The XOR for the range specified by the query is calculated using the prefix XOR array 'xr'. The result is pushed to the 'res' vector.
10 : Return Statement
return res;
The function returns the 'res' vector, which contains the results of all the XOR queries.
Best Case: O(n) - The best case occurs when all queries are processed after building the prefix XOR array.
Average Case: O(n + m) - Where n is the length of the array and m is the number of queries.
Worst Case: O(n + m) - The worst case involves processing all queries with the prefix XOR array.
Description: The time complexity is O(n + m), where n is the length of the array and m is the number of queries.
Best Case: O(n) - The best case still requires space for the prefix XOR array.
Worst Case: O(n) - The space complexity is O(n) due to the storage of the prefix XOR array.
Description: The space complexity is O(n), where n is the length of the array.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus