Leetcode 1302: Deepest Leaves Sum
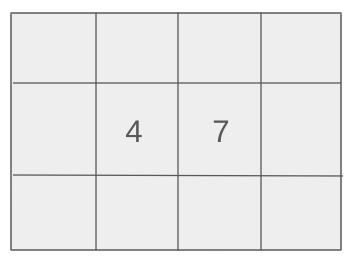
Given the root of a binary tree, return the sum of values of its deepest leaves. The deepest leaves are the nodes found at the lowest level of the tree.
Problem
Approach
Steps
Complexity
Input: The input consists of a binary tree where each node is represented by a value.
Example: Input: root = [10, 5, 15, 3, 7, null, 20, null, null, 18]
Constraints:
• The number of nodes in the tree is in the range [1, 10^4].
• 1 <= Node.val <= 100
Output: Return an integer representing the sum of the values of the deepest leaves in the binary tree.
Example: Output: 38
Constraints:
• The sum of the values of the deepest leaves.
Goal: To calculate the sum of the values of the deepest leaves of the binary tree.
Steps:
• Perform a level-order traversal (breadth-first search) of the binary tree to find the deepest level.
• At each level, accumulate the values of all nodes. The sum of the last level's nodes will be the sum of the deepest leaves.
Goal: The solution must be optimized to handle binary trees with up to 10^4 nodes efficiently.
Steps:
• 1 <= Number of nodes <= 10^4
• Node values range from 1 to 100.
Assumptions:
• The input tree is valid and non-empty.
• The number of nodes in the tree will always be within the specified range.
• Input: Input: root = [10, 5, 15, 3, 7, null, 20, null, null, 18]
• Explanation: The deepest leaves are 18 and 20. Their sum is 38.
• Input: Input: root = [12, 8, 20, 4, 10, 16, 25]
• Explanation: The deepest leaves are 16 and 25. Their sum is 41.
Approach: The optimal approach involves performing a level-order traversal of the tree to access all levels. By iterating through each level, we can find the deepest level's nodes and sum their values.
Observations:
• The deepest leaves are located at the last level of the tree. We need to find the last level efficiently.
• A breadth-first search is ideal for this problem, as it explores all nodes level by level.
Steps:
• Initialize a queue to perform a level-order traversal of the tree.
• For each level, keep track of the nodes in that level and their sum.
• Continue the traversal until you reach the last level, and return the sum of the nodes at that level.
Empty Inputs:
• The input tree will not be empty, as the problem guarantees that there is at least one node.
Large Inputs:
• For very large trees (up to 10^4 nodes), the solution must be optimized to avoid timeouts.
Special Values:
• If all nodes are at the same level, the sum will be the sum of all nodes in the tree.
Constraints:
• The solution must run in O(n) time where n is the number of nodes in the tree.
int deepestLeavesSum(TreeNode* root) {
if(root == NULL) return 0;
queue<TreeNode*> q;
q.push(root);
int sum = 0;
while(!q.empty()) {
int sz = q.size();
sum = 0;
while(sz--) {
sum += q.front()->val;
TreeNode* x = q.front();
q.pop();
if(x->left) q.push(x->left);
if(x->right) q.push(x->right);
}
}
return sum;
}
1 : Function Definition
int deepestLeavesSum(TreeNode* root) {
This line defines the function 'deepestLeavesSum' that takes the root of a binary tree ('root') as input and returns the sum of the values of the deepest leaves.
2 : Base Case Check
if(root == NULL) return 0;
Checks if the root is NULL (empty tree). If the tree is empty, it immediately returns 0 as there are no leaves to sum.
3 : Queue Initialization
queue<TreeNode*> q;
Declares a queue to facilitate a level-order traversal (breadth-first search) of the tree.
4 : Enqueue Root
q.push(root);
Pushes the root node into the queue to start the level-order traversal.
5 : Variable Initialization
int sum = 0;
Initializes the 'sum' variable to 0. This will accumulate the sum of values of nodes at the deepest level.
6 : While Loop - Tree Traversal
while(!q.empty()) {
Begins a while loop that continues as long as there are nodes in the queue to process.
7 : Queue Size
int sz = q.size();
Gets the size of the queue, which represents the number of nodes at the current level of the tree.
8 : Sum Reset
sum = 0;
Resets the 'sum' to 0 for each level of the tree, ensuring only the sum of the current level's nodes is calculated.
9 : Inner While Loop - Node Processing
while(sz--) {
Processes each node at the current level by iterating over the queue until all nodes at this level have been visited.
10 : Summing Node Values
sum += q.front()->val;
Adds the value of the node at the front of the queue to the 'sum' variable.
11 : Pop Front Node
TreeNode* x = q.front();
Stores the front node of the queue in variable 'x' to process it.
12 : Remove Processed Node
q.pop();
Removes the front node from the queue after processing it.
13 : Enqueue Left Child
if(x->left) q.push(x->left);
Checks if the current node has a left child. If so, it adds the left child to the queue.
14 : Enqueue Right Child
if(x->right) q.push(x->right);
Checks if the current node has a right child. If so, it adds the right child to the queue.
15 : Return Deepest Sum
return sum;
Returns the sum of the node values at the deepest level of the tree, which was accumulated in the 'sum' variable.
Best Case: O(n) - In the best case, the traversal will visit all nodes once.
Average Case: O(n) - On average, we will still visit all nodes once during the level-order traversal.
Worst Case: O(n) - In the worst case, we visit every node in the tree.
Description: The time complexity is O(n), where n is the number of nodes in the binary tree.
Best Case: O(n) - In the best case, the queue will hold all the nodes at the deepest level.
Worst Case: O(n) - Space complexity is O(n) due to the storage requirements for the queue during the level-order traversal.
Description: The space complexity is O(n), where n is the number of nodes in the tree.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus