Leetcode 1300: Sum of Mutated Array Closest to Target
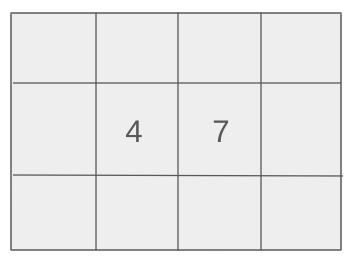
Given an integer array
arr
and a target value target
, return the integer value such that when all integers larger than this value are replaced by the value itself, the sum of the array is as close as possible to the target. If there is a tie, return the smallest such integer.Problem
Approach
Steps
Complexity
Input: The input consists of an integer array `arr` and an integer `target`.
Example: Input: arr = [5, 10, 3], target = 12
Constraints:
• 1 <= arr.length <= 10^4
• 1 <= arr[i], target <= 10^5
Output: Return the integer value that minimizes the absolute difference between the sum of the modified array and the target value.
Example: Output: 3
Constraints:
• Return an integer representing the optimal value.
Goal: Find the optimal integer value for modifying the array to match the target sum as closely as possible.
Steps:
• Sort the array `arr` to make the process of finding the best value more efficient.
• Calculate the sum of the array and adjust the larger values to be equal to the current candidate value.
• Track the closest sum to the target, and in case of a tie, return the smallest value.
Goal: Handle large input sizes efficiently while ensuring the modified array's sum is as close as possible to the target.
Steps:
• 1 <= arr.length <= 10^4
• 1 <= arr[i], target <= 10^5
Assumptions:
• The array `arr` consists of positive integers.
• The input is valid, and constraints are adhered to.
• Input: Input: arr = [5, 10, 3], target = 12
• Explanation: When using the value 3, the array becomes [3, 3, 3], which gives a sum of 9. This is the closest sum to the target of 12.
• Input: Input: arr = [1, 2, 3], target = 6
• Explanation: Using the value 2, the array becomes [2, 2, 2], which exactly matches the target sum of 6.
• Input: Input: arr = [100, 200, 300], target = 350
• Explanation: Using the value 150, the array becomes [150, 150, 150], which gives a sum of 450. This is the closest sum to the target of 350.
Approach: To solve this problem efficiently, we can consider sorting the array and finding the best integer by modifying the array's sum iteratively. This approach ensures that we calculate the closest sum without having to examine all possible values exhaustively.
Observations:
• The array's sum will be affected by replacing values larger than a given candidate with that candidate.
• Sorting the array will allow for efficient modification of the array as we check each potential candidate.
• We can use a greedy approach, adjusting values larger than the candidate and tracking the sum's proximity to the target.
Steps:
• Sort the array to make it easier to modify values larger than the current candidate.
• For each potential value (candidate), compute the sum of the modified array.
• Track the difference between the modified sum and the target. Return the candidate that minimizes this difference.
Empty Inputs:
• The problem guarantees that `arr` will contain at least one element.
Large Inputs:
• The solution must handle large arrays efficiently, ensuring time complexity remains manageable.
Special Values:
• If all values in the array are larger than the target, adjust all values to be equal to the target.
Constraints:
• The solution must work within the time limits for inputs of size up to 10^4.
int findBestValue(vector<int>& A, int target) {
sort(A.begin(), A.end());
int n = A.size(), i = 0;
while(i < n && target > A[i] * (n - i)) {
target -= A[i++];
}
if (i == n) return A[n - 1];
int res = target / (n - i);
if (target - res * (n - i) > (res + 1) * (n - i) - target)
res++;
return res;
}
1 : Function Definition
int findBestValue(vector<int>& A, int target) {
This is the function header where the function is defined. The input parameters are 'A' (a vector of integers) and 'target' (the target sum to be achieved).
2 : Sorting
sort(A.begin(), A.end());
Sorts the input vector 'A' in non-decreasing order. Sorting helps in efficiently calculating the optimal value to achieve the target sum.
3 : Size and Initialization
int n = A.size(), i = 0;
Initializes 'n' with the size of array 'A' and 'i' to 0 to start iterating from the beginning of the array.
4 : While Loop
while(i < n && target > A[i] * (n - i)) {
This while loop continues reducing the target by subtracting elements from 'A' until the remaining target cannot be further reduced by multiplying the current element with the remaining number of elements.
5 : Target Update
target -= A[i++];
This line reduces the target by subtracting the current element 'A[i]' and then increments 'i' to move to the next element.
6 : Condition Check
if (i == n) return A[n - 1];
Checks if all elements have been processed. If 'i' equals 'n', it means the target is achieved or exhausted, and the last element of the array 'A[n-1]' is returned.
7 : Intermediate Result Calculation
int res = target / (n - i);
Calculates the intermediate result 'res' by dividing the remaining target by the number of elements left after 'i'. This gives an estimate of the value to set for the remaining elements.
8 : Condition for Increment
if (target - res * (n - i) > (res + 1) * (n - i) - target)
Checks if the difference between the current target and the product of 'res' and the number of remaining elements is greater than the difference between '(res + 1)' and the target. If so, it indicates that increasing 'res' will yield a value closer to the target.
9 : Increment Result
res++;
If the above condition is true, increment 'res' by 1 to bring it closer to the optimal value.
10 : Return Final Result
return res;
Returns the computed value 'res' which is the optimal value that makes the sum closest to the target.
Best Case: O(n log n) - Sorting the array is the most time-consuming operation.
Average Case: O(n log n) - Sorting dominates the complexity.
Worst Case: O(n log n) - Sorting and the subsequent evaluation for each candidate value.
Description: The time complexity is O(n log n) due to sorting the array.
Best Case: O(n) - Space complexity remains O(n) for the sorted array.
Worst Case: O(n) - Space complexity is O(n) for storing the sorted array.
Description: The space complexity is O(n) due to storing the array after sorting.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus