Leetcode 1297: Maximum Number of Occurrences of a Substring
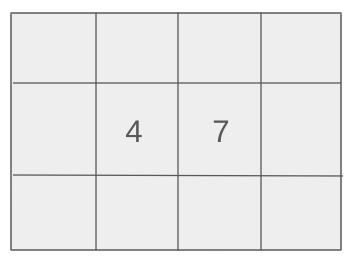
Given a string
s
, return the maximum number of occurrences of any substring that satisfies the following conditions: The number of unique characters in the substring must be less than or equal to maxLetters
, and the substring length must be between minSize
and maxSize
inclusive.Problem
Approach
Steps
Complexity
Input: The input consists of a string `s` and three integers: `maxLetters`, `minSize`, and `maxSize`.
Example: Input: s = "abcabcabc", maxLetters = 2, minSize = 2, maxSize = 3
Constraints:
• 1 <= s.length <= 10^5
• 1 <= maxLetters <= 26
• 1 <= minSize <= maxSize <= min(26, s.length)
Output: Return the maximum number of occurrences of any substring that satisfies the given conditions.
Example: Output: 2
Constraints:
• Return an integer representing the maximum number of occurrences of a valid substring.
Goal: Find the maximum number of occurrences of a valid substring.
Steps:
• Generate all substrings of length between `minSize` and `maxSize`.
• For each substring, check if it has unique characters less than or equal to `maxLetters`.
• Count the occurrences of valid substrings and track the maximum count.
Goal: The algorithm must handle large input sizes efficiently and adhere to the given constraints.
Steps:
• 1 <= s.length <= 10^5
• 1 <= maxLetters <= 26
• 1 <= minSize <= maxSize <= min(26, s.length)
Assumptions:
• The string `s` consists of lowercase English letters only.
• The input is valid, and constraints are adhered to.
• Input: Input: s = "abcabcabc", maxLetters = 2, minSize = 2, maxSize = 3
• Explanation: The substring 'ab' appears twice in the string and satisfies the condition of having 2 unique letters and a size of 2.
• Input: Input: s = "xxxyyy", maxLetters = 1, minSize = 2, maxSize = 3
• Explanation: The substring 'xx' appears twice and satisfies the condition of having 1 unique letter and a size of 2.
Approach: The problem can be solved by generating all possible substrings of valid lengths and counting their occurrences. We need to ensure that only valid substrings are considered.
Observations:
• We need to generate substrings of different lengths between `minSize` and `maxSize`.
• The number of unique characters in each substring must be tracked to check if it is valid.
• A sliding window approach or hashing can be used to efficiently count occurrences of substrings while ensuring they meet the conditions.
Steps:
• Iterate through all possible substring lengths from `minSize` to `maxSize`.
• For each substring, track the unique characters using a set or hash map.
• If the number of unique characters is less than or equal to `maxLetters`, update the count for that substring.
• After processing all substrings, return the maximum count of any valid substring.
Empty Inputs:
• The string is non-empty, as per the problem constraints.
Large Inputs:
• The solution must efficiently handle strings up to length 10^5.
Special Values:
• If `maxLetters` is larger than the number of unique letters in the string, all substrings are valid.
Constraints:
• The solution should be optimized to run within time limits for large input sizes.
bool isPossibleDivide(vector<int>& nums, int k) {
map<int, int> cnt;
int n = nums.size();
for(int num : nums)
cnt[num]++;
for(auto it : cnt) {
int frq = it.second;
if(frq > 0)
for(int i = 0; i < k; i++) {
if(cnt[it.first + i] < frq) return false;
else cnt[it.first + i] -= frq;
}
}
return true;
}
1 : Function Definition
bool isPossibleDivide(vector<int>& nums, int k) {
This is the function header, where the function is defined with two parameters: 'nums' (a vector of integers) and 'k' (the size of each group).
2 : Map Initialization
map<int, int> cnt;
This creates a map to store the frequency of each integer in the input array 'nums'. The key is the integer, and the value is its frequency.
3 : Array Length
int n = nums.size();
This stores the size of the input array 'nums' in the variable 'n'.
4 : Iterate Through Numbers
for(int num : nums)
This loop iterates through each integer in the input array 'nums'.
5 : Update Frequency Map
cnt[num]++;
This increments the frequency of the current number in the map 'cnt'.
6 : Frequency Map Iteration
Now, we begin iterating through the frequency map to check if it is possible to form groups.
7 : Iterate Through Frequency Map
for(auto it : cnt) {
This loop iterates through each key-value pair in the frequency map, where 'it.first' is the number and 'it.second' is its frequency.
8 : Get Frequency of Current Number
int frq = it.second;
Extracts the frequency of the current number from the map.
9 : Frequency Check
if(frq > 0)
Checks if the current frequency is greater than 0 before attempting to form a group.
10 : Loop for Consecutive Numbers
for(int i = 0; i < k; i++) {
This inner loop iterates over the next 'k' consecutive numbers starting from the current number.
11 : Condition for Group Formation
Here, we check if the group of consecutive numbers can be formed.
12 : Check for Sufficient Frequency
if(cnt[it.first + i] < frq) return false;
If the frequency of any of the consecutive numbers is less than the required frequency, it returns false as the group cannot be formed.
13 : Update Frequency for Used Numbers
else cnt[it.first + i] -= frq;
If the consecutive number can be used, its frequency is decreased by 'frq'.
14 : Return Statement
return true;
If the loop completes without returning false, it means it's possible to divide the array into consecutive groups of size 'k', so we return true.
Best Case: O(n) - In the best case, no substrings need to be checked or all substrings are valid.
Average Case: O(n^2) - Substring generation and uniqueness checks dominate the complexity.
Worst Case: O(n^2) - In the worst case, we need to check all possible substrings for validity.
Description: The time complexity is O(n^2) due to the need to generate and check all substrings.
Best Case: O(n) - Space complexity remains O(n) even in the best case.
Worst Case: O(n) - Space complexity is O(n) due to storage of substring counts and temporary sets.
Description: The space complexity is O(n) due to the temporary storage of unique substrings.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus