Leetcode 1296: Divide Array in Sets of K Consecutive Numbers
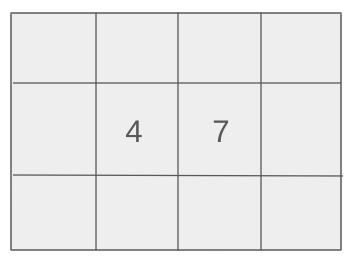
Given an array of integers
nums
and a positive integer k
, check if it is possible to divide the array into sets of k
consecutive numbers.Problem
Approach
Steps
Complexity
Input: The input consists of an integer array `nums` and a positive integer `k`.
Example: Input: nums = [5, 1, 2, 3, 4, 3, 6, 4], k = 4
Constraints:
• 1 <= k <= nums.length <= 10^5
• 1 <= nums[i] <= 10^9
Output: The function should return `true` if it's possible to divide the array into sets of `k` consecutive numbers, and `false` otherwise.
Example: Output: true
Constraints:
• Return a boolean value.
Goal: Determine if it is possible to divide the array into sets of `k` consecutive numbers.
Steps:
• Count the frequency of each number in the array using a map.
• For each number, check if a consecutive sequence starting from that number can be formed by checking its next `k-1` consecutive elements.
• If a number is used in a valid sequence, reduce its frequency in the map.
• Return `true` if all numbers are successfully grouped into sets of `k` consecutive numbers, otherwise return `false`.
Goal: Ensure that the algorithm handles large arrays and integers efficiently.
Steps:
• 1 <= k <= nums.length <= 10^5
• 1 <= nums[i] <= 10^9
Assumptions:
• The array `nums` contains integers and is not empty.
• The value of `k` is positive and less than or equal to the size of the array.
• Input: Input: nums = [5, 1, 2, 3, 4, 3, 6, 4], k = 4
• Explanation: The array can be grouped into the sets [1, 2, 3, 4] and [3, 4, 5, 6], so the output is `true`.
• Input: Input: nums = [2, 3, 4, 1], k = 3
• Explanation: It's not possible to divide the array into sets of 3 consecutive numbers, so the output is `false`.
Approach: This problem can be solved by using a frequency map and trying to form consecutive sequences starting from the smallest available number.
Observations:
• We need to efficiently count and group elements to form consecutive sequences.
• Using a map to track element frequencies will help us in forming the sets.
• By reducing the frequency of elements as we use them to form sets, we ensure that each number is part of exactly one set.
Steps:
• Count the frequency of each number in `nums` using a map.
• Sort the keys in the map to start from the smallest number.
• For each key, check if we can form a set of `k` consecutive numbers starting from that number.
• If possible, reduce the frequency of the used numbers in the map.
• Return `true` if all numbers are grouped into sets, otherwise return `false`.
Empty Inputs:
• The input array is never empty according to the constraints.
Large Inputs:
• Ensure the solution is optimized for large arrays of size up to 10^5.
Special Values:
• If the number of elements in the array is less than `k`, it's impossible to form a valid set.
Constraints:
• The solution must handle large input sizes efficiently, with time complexity ideally around O(n log n).
bool isPossibleDivide(vector<int>& nums, int k) {
map<int, int> cnt;
int n = nums.size();
for(int num : nums)
cnt[num]++;
for(auto it : cnt) {
int frq = it.second;
if(frq > 0)
for(int i = 0; i < k; i++) {
if(cnt[it.first + i] < frq) return false;
else cnt[it.first + i] -= frq;
}
}
return true;
}
1 : Function Definition
bool isPossibleDivide(vector<int>& nums, int k) {
Defines a function that checks if it is possible to divide the input array into groups of 'k' consecutive integers.
2 : Frequency Calculation
map<int, int> cnt;
Creates a map to store the frequency of each integer in the array.
3 : Array Length
int n = nums.size();
Stores the size of the input array.
4 : For Each Element
for(int num : nums)
Iterates through each element of the input array.
5 : Frequency Update
cnt[num]++;
Increments the frequency count for each number in the map.
6 : For Each Frequency
for(auto it : cnt) {
Iterates through each number and its frequency in the map.
7 : Get Frequency
int frq = it.second;
Extracts the frequency of the current number.
8 : Check Frequency
if(frq > 0)
Checks if the frequency of the current number is greater than zero.
9 : Loop for Consecutive Numbers
for(int i = 0; i < k; i++) {
Iterates through the next 'k' consecutive numbers.
10 : Return False if Not Enough Numbers
if(cnt[it.first + i] < frq) return false;
If the frequency of any consecutive number is less than the required, returns false.
11 : Update Frequency
else cnt[it.first + i] -= frq;
Decreases the frequency of the current consecutive number as it is used in the group.
12 : Return True
return true;
Returns true if it is possible to divide the numbers into consecutive groups of size 'k'.
Best Case: O(n log n) - The best case occurs when the elements are already in the correct order and can be grouped quickly.
Average Case: O(n log n) - Sorting and grouping elements dominate the time complexity.
Worst Case: O(n log n) - The worst case is when sorting and grouping take the longest time.
Description: The time complexity is O(n log n) due to sorting the keys of the frequency map.
Best Case: O(n) - The space complexity remains O(n) even in the best case.
Worst Case: O(n) - The space complexity is O(n) because of the frequency map storing all elements.
Description: The space complexity is O(n) due to the frequency map used to track the elements.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus