Leetcode 1286: Iterator for Combination
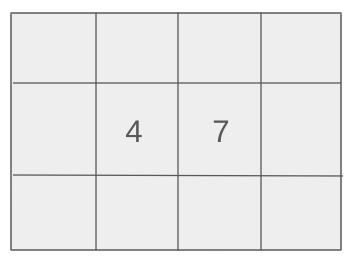
Design a CombinationIterator class that generates all combinations of a specified length from a sorted string of distinct lowercase English letters, and allows iterating through them in lexicographical order.
Problem
Approach
Steps
Complexity
Input: The input consists of a string of distinct lowercase English letters and an integer specifying the combination length.
Example: Input: characters = 'abc', combinationLength = 2
Constraints:
• 1 <= combinationLength <= characters.length <= 15
• All characters in characters are distinct.
Output: The iterator will provide the next combination when next() is called and will return true for hasNext() if there are more combinations left.
Example: Output: 'ab', true, 'ac', true, 'bc', false
Constraints:
• Each call to next() must return a valid combination.
Goal: The goal is to efficiently generate and return combinations of the specified length in lexicographical order.
Steps:
• Generate all combinations of the specified length from the string of characters.
• Store the combinations in a sequence.
• Use an index to iterate over the combinations, providing the next combination on each call to next().
• Check if there are more combinations available with the hasNext() function.
Goal: The constraints ensure that the number of combinations will not be excessive and can be handled efficiently.
Steps:
• 1 <= combinationLength <= 15
• The length of the string is at most 15.
• At most 10^4 calls to next() and hasNext() will be made.
Assumptions:
• The characters input is always sorted and contains distinct lowercase letters.
• Input: Input: characters = 'abc', combinationLength = 2
• Explanation: In this case, the combinations of length 2 are 'ab', 'ac', and 'bc'. The iterator will provide these combinations in order.
Approach: We can generate all combinations of the specified length from the given string, store them, and then return each combination one by one using an index.
Observations:
• Combinations should be generated in lexicographical order.
• A backtracking approach can be used to generate all combinations.
Steps:
• Implement a backtracking function to generate all combinations of the specified length.
• Store the generated combinations in a vector or list.
• Maintain an index to keep track of the current combination that is returned by next().
• Ensure that hasNext() checks if there are remaining combinations to iterate through.
Empty Inputs:
• If the input string is empty, the combinationLength must be 0, and no combinations can be generated.
Large Inputs:
• For very large input sizes (up to 15 characters), ensure the solution can handle generating and storing the combinations efficiently.
Special Values:
• When the combinationLength is equal to the length of the input string, the only possible combination is the entire string.
Constraints:
• Make sure the time complexity of generating combinations and iterating through them is optimized.
string str;
int len;
vector<string> ans;
map<int, int> mp;
void bt(int idx, string &tmp) {
if(tmp.size() == len) {
ans.push_back(tmp);
return;
}
if(idx == str.size()) {
return;
}
for(int i = idx; i < str.size(); i++) {
tmp += str[i];
bt(i + 1, tmp);
tmp.pop_back();
}
}
int idx = 0;
CombinationIterator(string chars, int len) {
this->len = len;
sort(chars.begin(), chars.end());
str = chars;
string tmp = "";
bt(0, tmp);
}
string next() {
return ans[idx++];
}
bool hasNext() {
if(idx < ans.size()) return true;
return false;
}
};
/**
* Your CombinationIterator object will be instantiated and called as such:
* CombinationIterator* obj = new CombinationIterator(characters, combinationLength);
* string param_1 = obj->next();
* bool param_2 = obj->hasNext();
1 : Variable Declaration
string str;
Declares a string to hold the input characters.
2 : Variable Declaration
int len;
Declares an integer to store the length of combinations.
3 : Variable Declaration
vector<string> ans;
Defines a vector to store the generated combinations.
4 : Data Structure
map<int, int> mp;
Defines a map, though it is unused in this context.
5 : Backtracking Function
void bt(int idx, string &tmp) {
Defines a recursive function for backtracking to generate combinations.
6 : Base Condition
if(tmp.size() == len) {
Checks if the current string has reached the desired length.
7 : Push to Results
ans.push_back(tmp);
Adds the completed combination to the results vector.
8 : Return Statement
return;
Exits the recursive call when a combination is completed.
9 : End Condition
if(idx == str.size()) {
Checks if the index has reached the end of the string.
10 : Return Statement
return;
Returns from the function if there are no more characters to explore.
11 : Loop Start
for(int i = idx; i < str.size(); i++) {
Loops through each character starting from the current index.
12 : Add Character
tmp += str[i];
Adds the current character to the temporary string.
13 : Recursive Call
bt(i + 1, tmp);
Recursively calls the backtracking function for the next index.
14 : Backtrack Step
tmp.pop_back();
Removes the last character to explore other combinations.
15 : Constructor Initialization
CombinationIterator(string chars, int len) {
Initializes the CombinationIterator with the sorted string and combination length.
16 : Assign Value
this->len = len;
Assigns the input length to the class variable.
17 : Sort Input
sort(chars.begin(), chars.end());
Sorts the input characters to ensure combinations are generated lexicographically.
18 : Set Input
str = chars;
Assigns the sorted characters to the class variable.
19 : Temporary String
string tmp = "";
Defines an empty temporary string for backtracking.
20 : Call Backtracking
bt(0, tmp);
Starts the backtracking process from the first index.
21 : Retrieve Next
string next() {
Defines a method to return the next combination.
22 : Increment Index
return ans[idx++];
Returns the current combination and increments the index.
23 : Check Availability
bool hasNext() {
Defines a method to check if more combinations are available.
24 : Return Condition
if(idx < ans.size()) return true;
Returns true if there are more combinations left to retrieve.
Best Case: O(1) - If there is only one combination to generate.
Average Case: O(n) - Generating and iterating through the combinations is linear with respect to the number of combinations.
Worst Case: O(n) - The worst case is when we have to generate and iterate through all combinations.
Description: The time complexity is determined by the need to generate and iterate through the combinations. Since there are n combinations, the complexity is O(n).
Best Case: O(1) - If no combinations are stored.
Worst Case: O(n) - Storing all combinations requires space proportional to the number of combinations.
Description: The space complexity is O(n), where n is the number of combinations generated, since each combination needs to be stored.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus