Leetcode 1283: Find the Smallest Divisor Given a Threshold
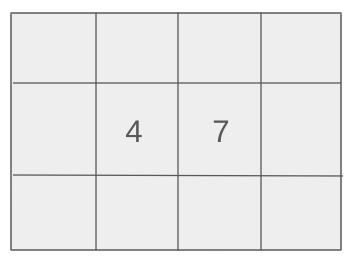
Given an array of integers and a threshold, find the smallest divisor such that the sum of the division results (rounded up) is less than or equal to the threshold.
Problem
Approach
Steps
Complexity
Input: You are given an array `nums` of integers and an integer `threshold`.
Example: Input: nums = [2,4,8,16], threshold = 8
Constraints:
• 1 <= nums.length <= 5 * 10^4
• 1 <= nums[i] <= 10^6
• nums.length <= threshold <= 10^6
Output: Return the smallest integer divisor such that the sum of the division results (rounded up) is less than or equal to the threshold.
Example: Output: 4
Constraints:
• The divisor must be a positive integer.
Goal: The goal is to find the smallest divisor such that the sum of the rounded-up division results is less than or equal to the threshold.
Steps:
• Initialize a binary search range from 1 to max(nums).
• For each divisor in the range, calculate the sum of rounded-up division results of each element in `nums`.
• Check if the sum is less than or equal to the threshold. If it is, narrow the search range to smaller divisors. If it is greater, narrow the range to larger divisors.
Goal: Ensure that the divisor satisfies the condition where the sum of division results is less than or equal to the threshold.
Steps:
• 1 <= nums.length <= 50,000
• 1 <= nums[i] <= 1,000,000
• 1 <= threshold <= 1,000,000
Assumptions:
• There will always be a valid solution for the given input.
• Input: Input: nums = [2,4,8,16], threshold = 8
• Explanation: In this example, we need to select a divisor such that the sum of the results of dividing each number in `nums` by the divisor is less than or equal to 8. The smallest divisor that satisfies this condition is 4.
Approach: We can use binary search to find the smallest divisor. The binary search will help efficiently narrow down the possible divisors.
Observations:
• We need to minimize the divisor while ensuring the sum of the division results remains below the threshold.
• Binary search is a suitable approach to minimize the divisor because the sum decreases as the divisor increases.
Steps:
• Set the left boundary of binary search to 1 and the right boundary to the maximum value in `nums`.
• For each mid-value in the binary search, calculate the sum of division results and check if it is less than or equal to the threshold.
• If the sum exceeds the threshold, increase the divisor. If the sum is within the threshold, reduce the divisor.
Empty Inputs:
• If `nums` is empty, return 1 as the divisor, but this case is not expected to occur based on the problem constraints.
Large Inputs:
• Ensure that the binary search approach works efficiently even for the largest inputs (i.e., `nums.length` up to 50,000).
Special Values:
• If all elements in `nums` are equal, the divisor will likely be close to the value of each element for an optimal sum.
Constraints:
• The approach should work within the problem's time and space limits.
int smallestDivisor(vector<int>& nums, int threshold) {
int left = 1, right = 1e6, m , sum;
while(left < right) {
m = (left + right) / 2;
sum = 0;
for(int i: nums) sum += (i + m - 1)/m;
if(sum > threshold)
left = m + 1;
else right = m;
}
return left;
}
1 : Function Definition
int smallestDivisor(vector<int>& nums, int threshold) {
Defines the function to find the smallest divisor meeting the threshold constraint.
2 : Variable Initialization
int left = 1, right = 1e6, m , sum;
Initializes the binary search bounds and other variables.
3 : Binary Search Loop
while(left < right) {
Starts the binary search loop to find the smallest valid divisor.
4 : Midpoint Calculation
m = (left + right) / 2;
Calculates the middle value between the binary search bounds.
5 : Reset Sum
sum = 0;
Resets the sum for the current divisor.
6 : Sum Calculation
for(int i: nums) sum += (i + m - 1)/m;
Calculates the sum of quotients for the current divisor.
7 : Condition Check
if(sum > threshold)
Checks if the sum exceeds the threshold.
8 : Update Left Bound
left = m + 1;
Increases the lower bound of the binary search if the condition is met.
9 : Update Right Bound
else right = m;
Decreases the upper bound of the binary search otherwise.
10 : Return Statement
return left;
Returns the smallest divisor that satisfies the threshold condition.
Best Case: O(n log(max(nums)))
Average Case: O(n log(max(nums)))
Worst Case: O(n log(max(nums)))
Description: The time complexity is O(n log(max(nums))) because we perform binary search (log(max(nums))) and for each iteration, we sum the results of dividing each element in `nums` (O(n)).
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the storage needed for the division results.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus