Leetcode 1276: Number of Burgers with No Waste of Ingredients
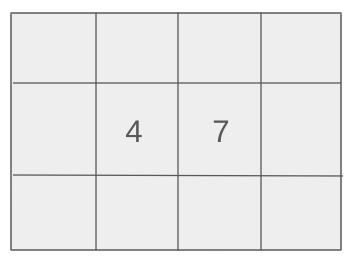
Given two integers tomatoSlices and cheeseSlices, return the number of Jumbo and Small burgers that can be made, such that all tomatoSlices and cheeseSlices are used. If it is not possible, return an empty list.
Problem
Approach
Steps
Complexity
Input: You are given two integers representing the number of tomato slices and cheese slices.
Example: Input: tomatoSlices = 16, cheeseSlices = 7
Constraints:
• 0 <= tomatoSlices, cheeseSlices <= 10^7
Output: Return a list with the number of Jumbo and Small burgers or an empty list if it is not possible.
Example: Output: [1, 6]
Constraints:
• The number of burgers must use all of the given slices.
Goal: Determine how many Jumbo and Small burgers can be made using all the given tomato and cheese slices.
Steps:
• Calculate the number of Jumbo burgers (which require 4 tomato slices and 1 cheese slice).
• Calculate the number of Small burgers (which require 2 tomato slices and 1 cheese slice).
• Check if the total number of ingredients can be used exactly without any leftovers.
Goal: The solution must handle inputs within the specified limits efficiently.
Steps:
• 0 <= tomatoSlices, cheeseSlices <= 10^7
• The number of burger combinations should be calculated optimally.
Assumptions:
• There are always positive integer values for tomatoSlices and cheeseSlices.
• Input: Input: tomatoSlices = 16, cheeseSlices = 7
• Explanation: To make one Jumbo burger and six Small burgers, the total number of tomato slices and cheese slices will match exactly with the given inputs.
Approach: The problem can be solved using a simple mathematical approach by calculating the number of burgers and checking if the total slices are used up.
Observations:
• Each Jumbo burger requires 4 tomato slices and 1 cheese slice.
• Each Small burger requires 2 tomato slices and 1 cheese slice.
• We can calculate how many Jumbo burgers can be made using the formula (tomatoSlices - 2 * cheeseSlices) / 2. Then, check if the rest of the slices can form Small burgers.
Steps:
• First, check if the remaining number of tomatoSlices after making Jumbo burgers is divisible by 2.
• Then, calculate the number of Small burgers and check if the total number of ingredients is used up correctly.
Empty Inputs:
• If either tomatoSlices or cheeseSlices is zero, no burgers can be made.
Large Inputs:
• For very large inputs, ensure that the solution can handle up to 10^7 efficiently.
Special Values:
• If the number of tomatoSlices and cheeseSlices cannot form a valid combination, return an empty list.
Constraints:
• Ensure that no negative values are passed for tomatoSlices and cheeseSlices.
vector<int> ans;
map<int, map<int, bool>> mp;
bool dp(int tmt, int chs, int one, int two) {
if(tmt <= 0 || chs <= 0) {
if(tmt == 0 && chs == 0) {
ans = {one, two};
return true;
}
return false;
}
if(mp.count(tmt) && mp[tmt].count(chs)) return mp[tmt][chs];
if(tmt >= 4 && chs >= 1) {
if(dp(tmt - 4, chs -1, one + 1, two))
return mp[tmt][chs] = true;
if(dp(tmt - 2, chs -1, one, two + 1))
return mp[tmt][chs] = true;
} else if(tmt >= 2 && chs >= 1) {
if(dp(tmt - 2, chs -1, one, two + 1))
return mp[tmt][chs] = true;
}
return mp[tmt][chs] = false;
}
vector<int> numOfBurgers(int tmt, int chs) {
ans = {};
// dp(tmt, chs, 0, 0);
int net = chs;
int jumbo = (tmt - 2 * chs ) / 2;
if(((tmt - 2 * chs ) < 0) || ((tmt - 2 * chs ) % 2) || (net - jumbo < 0)) return ans;
return {jumbo, net - jumbo};
}
1 : Variable Initialization
vector<int> ans;
Initialize a vector to store the results of the solution.
2 : Map Operations
map<int, map<int, bool>> mp;
Declare a nested map for memoization to store intermediate results for dynamic programming.
3 : Function Definition
bool dp(int tmt, int chs, int one, int two) {
Define a recursive function for dynamic programming to find the solution.
4 : Conditional Check
if(tmt <= 0 || chs <= 0) {
Check if the total or cheese constraints are invalid.
5 : Conditional Check
if(tmt == 0 && chs == 0) {
Verify if the constraints are perfectly met.
6 : Vector Insertion
ans = {one, two};
Assign the valid result to the answer vector.
7 : Return Statement
return true;
Indicate success when constraints are satisfied.
8 : Return Statement
return false;
Return false if constraints are not satisfied.
9 : Map Lookup
if(mp.count(tmt) && mp[tmt].count(chs)) return mp[tmt][chs];
Check if the result for the given state is already computed.
10 : Recursive Call
if(tmt >= 4 && chs >= 1) {
Check if sufficient tomatoes and cheese are available for a jumbo burger.
11 : Recursive Call
if(dp(tmt - 4, chs -1, one + 1, two))
Attempt to use a jumbo burger and recursively solve the remaining problem.
12 : Memoization Update
return mp[tmt][chs] = true;
Store the result in the memoization map and return success.
13 : Recursive Call
if(dp(tmt - 2, chs -1, one, two + 1))
Attempt to use a small burger and recursively solve the remaining problem.
14 : Memoization Update
return mp[tmt][chs] = true;
Store the result in the memoization map and return success.
15 : Return Statement
return mp[tmt][chs] = false;
Return false and update the memoization map for the given state.
16 : Function Definition
vector<int> numOfBurgers(int tmt, int chs) {
Define the main function to compute the number of burgers.
17 : Variable Initialization
ans = {};
Initialize the result vector as empty.
18 : Variable Initialization
int net = chs;
Calculate the net cheese count.
19 : Mathematical Operation
int jumbo = (tmt - 2 * chs ) / 2;
Compute the number of jumbo burgers using direct calculation.
20 : Conditional Check
if(((tmt - 2 * chs ) < 0) || ((tmt - 2 * chs ) % 2) || (net - jumbo < 0)) return ans;
Validate the constraints for feasible burger counts.
21 : Return Statement
return {jumbo, net - jumbo};
Return the calculated result with jumbo and small burgers.
Best Case: O(1)
Average Case: O(1)
Worst Case: O(1)
Description: The solution only requires a constant number of operations regardless of input size.
Best Case: O(1)
Worst Case: O(1)
Description: Only a constant amount of space is required.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus