Leetcode 1266: Minimum Time Visiting All Points
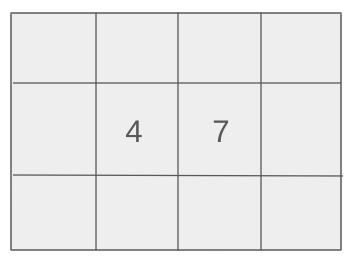
You are given an array of points with integer coordinates. Calculate the minimum time to visit all points in the given order using vertical, horizontal, or diagonal movements.
Problem
Approach
Steps
Complexity
Input: The input consists of an array of points, where each point is represented by a pair of integers [x, y].
Example: [[2, 2], [5, 5], [1, 3]]
Constraints:
• 1 <= points.length <= 100
• -1000 <= points[i][0], points[i][1] <= 1000
Output: The output is an integer representing the minimum time in seconds to visit all points in the given order.
Example: 6
Constraints:
• The time is measured in seconds, considering the movement options.
Goal: Determine the minimum time to visit all points in the given order.
Steps:
• 1. Calculate the time to move from the current point to the next using the maximum of the differences in x and y coordinates (taking diagonal moves into account).
• 2. Add the time for each pair of consecutive points to the total time.
• 3. Return the total time after visiting all points.
Goal: The constraints ensure that the solution works within the time and space limits.
Steps:
• 1 <= points.length <= 100
• -1000 <= points[i][0], points[i][1] <= 1000
Assumptions:
• The array contains at least one point.
• The input points are valid and within the given constraints.
• Input: [[2, 2], [5, 5], [1, 3]]
• Explanation: The total time to visit all points is 6 seconds: 3 seconds to go from [2, 2] to [5, 5] and 3 seconds to go from [5, 5] to [1, 3].
Approach: Use the concept of Manhattan distance but consider diagonal movements to minimize the time.
Observations:
• We can calculate the time for each move between points by considering the largest difference in coordinates (either horizontally or vertically).
• Diagonal moves can be used to reduce the total time.
• To find the minimum time, calculate the maximum of the horizontal and vertical distances between two consecutive points.
Steps:
• 1. Iterate through the list of points.
• 2. For each pair of consecutive points, calculate the time to move between them by taking the maximum of the absolute differences in x and y coordinates.
• 3. Add the time for each movement to the total time.
• 4. Return the total time after iterating through all points.
Empty Inputs:
• The input array will always contain at least one point as per the constraints.
Large Inputs:
• Ensure that the solution handles the maximum number of points efficiently.
Special Values:
• All points may be located at the same position, which would result in zero time.
Constraints:
• The input is valid and within the problem's constraints.
int minTimeToVisitAllPoints(vector<vector<int>>& points) {
int ans = 0;
for(int i = 1; i < points.size(); i++) {
ans += max(abs(points[i][1] - points[i - 1][1]), abs(points[i][0] - points[i - 1][0]));
}
return ans;
}
1 : Function Definition
int minTimeToVisitAllPoints(vector<vector<int>>& points) {
This defines the function `minTimeToVisitAllPoints`, which takes a 2D vector `points` representing a list of coordinates and returns the minimum time required to visit all points.
2 : Variable Initialization
int ans = 0;
This initializes an integer variable `ans` to 0, which will accumulate the total time needed to visit all the points.
3 : Loop (Iterate Through Points)
for(int i = 1; i < points.size(); i++) {
This loop iterates through the points starting from the second point (index 1) and compares each point with the previous one.
4 : Distance Calculation
ans += max(abs(points[i][1] - points[i - 1][1]), abs(points[i][0] - points[i - 1][0]));
This calculates the time it takes to move from the previous point to the current point. The time is the maximum of the vertical and horizontal distance between the points.
5 : Return Statement
return ans;
This returns the total accumulated time `ans`, which represents the minimum time required to visit all points.
Best Case: O(n), where n is the number of points.
Average Case: O(n) for each iteration over the points.
Worst Case: O(n), where n is the number of points.
Description: The time complexity is linear because we iterate over the list of points once.
Best Case: O(1), as no additional space is used other than for variables.
Worst Case: O(1), since only a constant amount of extra space is needed.
Description: The space complexity is constant since only a few variables are used to calculate the total time.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus