Leetcode 1237: Find Positive Integer Solution for a Given Equation
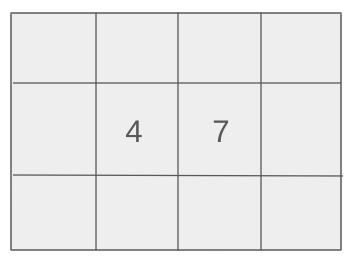
You are given a callable function f(x, y) with a hidden formula and a target value z. Your task is to find all pairs of positive integers x and y such that f(x, y) == z. The function is monotonically increasing, meaning: f(x, y) < f(x + 1, y) and f(x, y) < f(x, y + 1). Return a list of all such pairs. If no valid pairs are found, return an empty list.
Problem
Approach
Steps
Complexity
Input: The input consists of a function identifier and a target value z. The function_id will be used to choose the formula that defines f(x, y).
Example: Input: function_id = 1, z = 5
Constraints:
• 1 <= function_id <= 9
• 1 <= z <= 100
• 1 <= x, y <= 1000
Output: The output should be a list of pairs of positive integers [x, y] such that f(x, y) == z. If no such pairs exist, return an empty list.
Example: Output: [[1, 4], [2, 3], [3, 2], [4, 1]]
Constraints:
• The pairs should be ordered by x first, then by y.
Goal: The goal is to reverse-engineer the hidden formula and find all pairs (x, y) such that f(x, y) equals the target value z.
Steps:
• 1. Iterate over possible values of x and y within the defined range.
• 2. Use binary search to find the value of y for each x such that f(x, y) == z.
• 3. Collect and return all valid pairs.
Goal: The constraints on function_id and z are guaranteed to allow the solutions to fit in the valid range for x and y.
Steps:
• 1 <= function_id <= 9
• 1 <= z <= 100
• The function f(x, y) is monotonically increasing.
Assumptions:
• The function f(x, y) is deterministic and will return the same output for the same inputs every time.
• Input: Input: function_id = 1, z = 5
• Explanation: For function_id = 1, the formula is f(x, y) = x + y. The pairs [1, 4], [2, 3], [3, 2], [4, 1] all give f(x, y) = 5.
Approach: To solve this problem, we need to efficiently search for all valid pairs (x, y) where f(x, y) equals z using a binary search approach.
Observations:
• The function f(x, y) is monotonically increasing in both x and y.
• This allows us to apply binary search to reduce the search space.
• We can iterate through possible values of x and use binary search for y.
Steps:
• 1. Start with x = 1 and iterate up to 1000.
• 2. For each x, perform a binary search on y between 1 and 1000 to find where f(x, y) == z.
• 3. Collect all such pairs and return them.
Empty Inputs:
• If no valid pairs exist, the function should return an empty list.
Large Inputs:
• For larger values of z, the approach should efficiently search within the valid range of x and y.
Special Values:
• The approach should handle edge cases like the smallest and largest possible values of x and y.
Constraints:
• Ensure that the time complexity allows the solution to handle the maximum range of x and y (1 <= x, y <= 1000).
vector<vector<int>> findSolution(CustomFunction& cf, int z) {
int xl = 1, xr = 1000;
vector<vector<int>> res;
for(int x = 1; x <= 1000; x++) {
if(cf.f(x, 0) > z) break;
int ans = -1;
int yl = 1, yr = 1000;
while(yl <= yr) {
int ymid = yl + (yr - yl + 1) / 2;
int sol = cf.f(x, ymid);
if(sol == z) {
ans = ymid;
break;
}
if(sol < z) yl = ymid + 1;
else yr = ymid - 1;
}
if(ans != -1) res.push_back({x, ans});
}
return res;
}
1 : Function Definition
vector<vector<int>> findSolution(CustomFunction& cf, int z) {
The function `findSolution` is defined, which takes a `CustomFunction` object `cf` and an integer `z` as input and returns a 2D vector containing the pairs (x, y) where `f(x, y) == z`.
2 : Variable Initialization
int xl = 1, xr = 1000;
The variables `xl` and `xr` are initialized to 1 and 1000, respectively, representing the search range for the `x` values.
3 : Result Initialization
vector<vector<int>> res;
A 2D vector `res` is initialized to store the pairs (x, y) that satisfy the condition `f(x, y) == z`.
4 : Outer Loop
for(int x = 1; x <= 1000; x++) {
An outer loop starts that iterates over all possible values of `x` from 1 to 1000.
5 : Early Termination Check
if(cf.f(x, 0) > z) break;
If the result of `f(x, 0)` is greater than `z`, the loop is broken early as no valid pairs can be found for this `x`.
6 : Binary Search Initialization
int ans = -1;
The variable `ans` is initialized to -1, indicating that no valid `y` has been found yet for the current `x`.
7 : Binary Search Boundaries
int yl = 1, yr = 1000;
The binary search range for `y` is initialized with `yl = 1` and `yr = 1000`.
8 : Binary Search Loop
while(yl <= yr) {
A while loop begins to perform binary search within the range of `y` values from `yl` to `yr`.
9 : Binary Search Midpoint
int ymid = yl + (yr - yl + 1) / 2;
The midpoint `ymid` of the current `y` range is calculated using the formula `yl + (yr - yl + 1) / 2`.
10 : Function Evaluation
int sol = cf.f(x, ymid);
The function `f(x, ymid)` is evaluated to get the result `sol` for the current `x` and `ymid`.
11 : Solution Found
if(sol == z) {
If the result of `f(x, ymid)` is equal to `z`, the solution has been found for this pair (x, ymid).
12 : Store Solution
ans = ymid;
The variable `ans` is updated with the value of `ymid`, which is the value of `y` that satisfies `f(x, y) == z`.
13 : Break from Binary Search
break;
The loop breaks because a valid `y` has been found for the current `x`.
14 : Adjust Search Range
if(sol < z) yl = ymid + 1;
If `sol` is less than `z`, the search range for `y` is adjusted by setting `yl` to `ymid + 1` to search in the upper half.
15 : Adjust Search Range
else yr = ymid - 1;
If `sol` is greater than `z`, the search range for `y` is adjusted by setting `yr` to `ymid - 1` to search in the lower half.
16 : Store Valid Pair
if(ans != -1) res.push_back({x, ans});
If a valid `y` has been found (`ans != -1`), the pair (x, ans) is added to the result vector `res`.
17 : Return Result
return res;
The function returns the vector `res` containing all valid pairs (x, y) where `f(x, y) == z`.
Best Case: O(n log n), where n is the number of possible values of y for each x.
Average Case: O(n log n), where n is the number of values for y.
Worst Case: O(n log n), where n is the number of values for x and y.
Description: The time complexity is logarithmic for each binary search, and linear for iterating through x.
Best Case: O(1), if no valid pairs are found.
Worst Case: O(n), where n is the number of valid pairs.
Description: The space complexity is determined by the number of valid pairs returned.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus