Leetcode 1131: Maximum of Absolute Value Expression
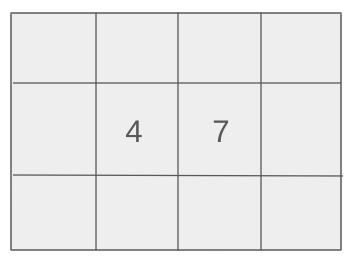
You are given two arrays arr1 and arr2 of integers, both having equal lengths. Calculate the maximum value of the expression: |arr1[i] - arr1[j]| + |arr2[i] - arr2[j]| + |i - j| for all pairs of indices i and j, where 0 <= i, j < arr1.length.
Problem
Approach
Steps
Complexity
Input: You are given two arrays arr1 and arr2 of integers of equal length.
Example: Input: arr1 = [3, 1, 4, 5], arr2 = [2, 0, 3, 4]
Constraints:
• 2 <= arr1.length == arr2.length <= 40000
• -10^6 <= arr1[i], arr2[i] <= 10^6
Output: Return the maximum value of the expression: |arr1[i] - arr1[j]| + |arr2[i] - arr2[j]| + |i - j|.
Example: Output: 9
Constraints:
• The answer fits within a 32-bit signed integer.
Goal: The goal is to compute the maximum value of the expression |arr1[i] - arr1[j]| + |arr2[i] - arr2[j]| + |i - j| for all pairs of indices.
Steps:
• Iterate through the array using nested loops for indices i and j.
• For each pair of indices, calculate the expression value and update the result if it's larger than the current maximum value.
Goal: Ensure the solution handles arrays of large sizes and values efficiently.
Steps:
• The array lengths must be between 2 and 40,000.
• The values of arr1 and arr2 are within the range [-10^6, 10^6].
Assumptions:
• The arrays are guaranteed to have the same length, and there will always be at least two elements.
• Input: Input: arr1 = [3, 1, 4, 5], arr2 = [2, 0, 3, 4]
• Explanation: For this example, the maximum value of the expression occurs at indices i = 0 and j = 3. The value of the expression is 9.
• Input: Input: arr1 = [1, -2, 3], arr2 = [0, 2, 1]
• Explanation: For this example, the maximum value occurs at indices i = 0 and j = 2. The value of the expression is 8.
Approach: The solution involves iterating through all pairs of indices and computing the value of the expression for each pair to find the maximum value.
Observations:
• We need to evaluate the expression for every possible pair of indices in the arrays.
• Considering the constraints, a brute force solution might be inefficient. We need to think of ways to optimize the approach.
Steps:
• Initialize a variable to track the maximum value of the expression.
• Use two nested loops to iterate through all pairs of indices i and j.
• For each pair, calculate the value of the expression and update the maximum value accordingly.
Empty Inputs:
• The problem guarantees that there will always be at least two elements in each array.
Large Inputs:
• The solution should be efficient enough to handle the largest input sizes (up to 40,000 elements).
Special Values:
• Consider arrays where all elements are the same or have maximum and minimum values, as this may affect the result.
Constraints:
• Ensure that the solution works efficiently within the provided constraints.
int maxAbsValExpr(vector<int>& x, vector<int>& y) {
int res = 0, n = x.size(), smallest, cur;
for(int p: {1, -1}) {
for(int q: {1, -1}) {
smallest = p * x[0] + q * y[0] + 0;
for(int i = 1; i < n; i++) {
cur = p * x[i] + q * y[i] + i;
res = max(res, cur - smallest);
smallest = min(smallest, cur);
}
}
}
return res;
}
1 : Function Definition
int maxAbsValExpr(vector<int>& x, vector<int>& y) {
This line defines the function `maxAbsValExpr`, which takes two integer arrays `x` and `y` and returns the maximum absolute value expression computed from them.
2 : Variable Initialization
int res = 0, n = x.size(), smallest, cur;
The variables `res` (to store the result), `n` (the size of the input arrays), `smallest` (to track the smallest computed expression), and `cur` (to store the current expression value) are initialized.
3 : Outer Loop
for(int p: {1, -1}) {
This outer `for` loop iterates over two values, `1` and `-1`, representing the possible signs to apply to the elements of array `x`.
4 : Inner Loop
for(int q: {1, -1}) {
The inner `for` loop iterates over two values, `1` and `-1`, representing the possible signs to apply to the elements of array `y`.
5 : Initial Expression Calculation
smallest = p * x[0] + q * y[0] + 0;
The initial expression for the first pair of elements from `x` and `y` is computed and assigned to `smallest`, where the sign for `x[0]` is determined by `p` and the sign for `y[0]` is determined by `q`.
6 : Loop Over Remaining Elements
for(int i = 1; i < n; i++) {
This `for` loop iterates over the remaining elements of the arrays `x` and `y` starting from index `1`.
7 : Current Expression Calculation
cur = p * x[i] + q * y[i] + i;
The current expression value `cur` is computed for each element of the arrays `x` and `y` with the corresponding sign applied based on `p` and `q`, and adding the index `i`.
8 : Update Result
res = max(res, cur - smallest);
The result `res` is updated with the maximum value between the current result and the difference between `cur` and `smallest`.
9 : Update Smallest Value
smallest = min(smallest, cur);
The variable `smallest` is updated to store the minimum value between `smallest` and the current expression value `cur`.
10 : Return Statement
return res;
The final result `res` is returned, which contains the maximum absolute value expression.
Best Case: O(n^2)
Average Case: O(n^2)
Worst Case: O(n^2)
Description: The time complexity is O(n^2) due to the nested iteration over all pairs of indices.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) as the solution only requires a constant amount of extra space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus