Leetcode 1111: Maximum Nesting Depth of Two Valid Parentheses Strings
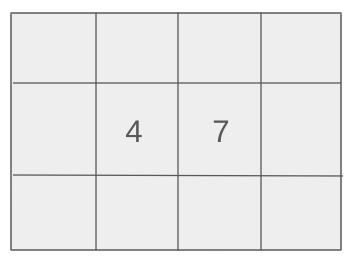
A string consisting of only ‘(’ and ‘)’ characters is considered a valid parentheses string (VPS) if it satisfies the following conditions: it is empty, can be split into two valid VPS’s, or can be written in the form of ‘(A)’ where A is a VPS. You are given a VPS string
seq
. The task is to split it into two subsequences, A and B, such that both A and B are valid parentheses strings. The goal is to minimize the maximum nesting depth between A and B, and you need to return an array where each element is 0 if the character is part of A, and 1 if it’s part of B.Problem
Approach
Steps
Complexity
Input: The input is a string `seq` consisting only of '(' and ')' characters representing a valid parentheses string.
Example: Input: seq = '(())(())'
Constraints:
• 1 <= seq.size <= 10000
Output: The output is an array of length `seq.length`, where each element is 0 if the character at that index is part of subsequence A, and 1 if it is part of subsequence B.
Example: Output: [0, 1, 0, 0, 1, 1, 0, 1]
Constraints:
• The array should satisfy the condition that both subsequences are valid parentheses strings.
Goal: The goal is to split the given VPS string into two subsequences, A and B, while minimizing the maximum depth of the subsequences.
Steps:
• Initialize a variable `d` to track the current depth of parentheses.
• Iterate through the string and for each character, increment or decrement `d` based on whether the character is '(' or ')'.
• For each character, assign it to subsequence A (0) or B (1) based on the parity of the current depth.
• Return the array representing the split subsequences.
Goal: The input string will be a valid parentheses string of length between 1 and 10,000.
Steps:
• 1 <= seq.size <= 10000
• The input string contains only '(' and ')' characters.
Assumptions:
• The input string is guaranteed to be a valid parentheses string.
• Input: Input: seq = '(())(())'
• Explanation: The optimal split would be into subsequences A = '(())' and B = '(())', where the resulting depths are both 1. The answer array could be [0, 1, 0, 0, 1, 1, 0, 1].
• Input: Input: seq = '(()())()'
• Explanation: An optimal split would be into subsequences A = '(())' and B = '()()', where the depths are 1 and 1, respectively. The answer array could be [0, 1, 1, 0, 0, 1, 1, 1].
Approach: We will split the string into two subsequences by iterating through it and assigning characters to subsequences A and B based on their nesting depth. This will minimize the maximum depth of both subsequences.
Observations:
• We can use a greedy approach to assign characters to subsequences while keeping track of the nesting depth.
• The depth of a parentheses string can be tracked as we traverse it, and we can alternate between subsequences based on this depth.
Steps:
• Initialize an empty list `groups` to store the answer array.
• Track the current depth `d` while iterating through the string.
• For each character, if it is '(', increment the depth; if it is ')', decrement the depth.
• Assign each character to subsequence A (0) or B (1) based on whether the current depth is odd or even.
• Return the `groups` list as the answer array.
Empty Inputs:
• The input will never be empty because it is guaranteed to be a valid parentheses string.
Large Inputs:
• The solution should efficiently handle inputs up to the size limit of 10,000 characters.
Special Values:
• A string with alternating parentheses like '()()()' will split evenly between A and B, maintaining the balance.
Constraints:
• Ensure that the solution adheres to the problem constraints.
vector<int> maxDepthAfterSplit(string seq) {
vector<int> groups;
int d = 0;
for(char c: seq) {
bool open = c == '(';
if(open) d++;
groups.push_back(d%2);
if(!open) d--;
}
return groups;
}
1 : Function Declaration
vector<int> maxDepthAfterSplit(string seq) {
The function `maxDepthAfterSplit` is defined, taking a string `seq` representing a sequence of parentheses. It returns a vector of integers representing the depth of each parenthesis in two alternating groups.
2 : Variable Declaration
vector<int> groups;
A vector `groups` is declared to store the resulting depth group (0 or 1) for each parenthesis in the sequence.
3 : Variable Declaration
int d = 0;
An integer `d` is initialized to 0, which will track the current depth of the parentheses during traversal.
4 : Loop
for(char c: seq) {
A `for` loop begins to iterate over each character `c` in the input string `seq`.
5 : Boolean Check
bool open = c == '(';
A boolean variable `open` is set to `true` if the character `c` is an opening parenthesis ('('), otherwise it is `false`.
6 : Condition Check
if(open) d++;
If the current character is an opening parenthesis, the depth `d` is incremented.
7 : Vector Operation
groups.push_back(d%2);
The current depth modulo 2 (`d%2`) is added to the `groups` vector, ensuring alternating group assignment (0 or 1) for each level of depth.
8 : Condition Check
if(!open) d--;
If the current character is a closing parenthesis, the depth `d` is decremented.
9 : Return Statement
return groups;
The function returns the `groups` vector, which contains the alternating depth groups (0 or 1) for each parenthesis in the sequence.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) because we traverse the string exactly once.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) because we store the result array of the same length as the input string.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus