Leetcode 1054: Distant Barcodes
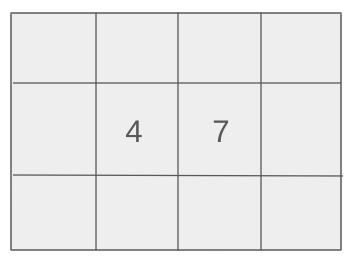
You are given an array of barcodes where each element represents a barcode. Your task is to rearrange the barcodes such that no two adjacent barcodes are the same. It is guaranteed that a solution exists, and you may return any valid rearrangement.
Problem
Approach
Steps
Complexity
Input: You are given an array of integers, where each element represents a barcode. The goal is to rearrange these integers such that no two adjacent integers are the same.
Example: Input: barcodes = [4, 4, 7, 7, 8, 8]
Constraints:
• 1 <= barcodes.length <= 10,000
• 1 <= barcodes[i] <= 10,000
Output: The output should be an array of integers, where no two adjacent integers are the same. The arrangement should be a valid permutation of the input array.
Example: Output: [7, 4, 7, 4, 8, 8]
Constraints:
• The output must be a valid permutation of the input array.
Goal: The goal is to rearrange the given barcodes such that no two adjacent barcodes are identical.
Steps:
• 1. Count the frequency of each barcode in the array.
• 2. Use a greedy approach to place the most frequent barcode in the array first, alternating the placement to ensure no two adjacent barcodes are the same.
• 3. Fill in the remaining barcodes, ensuring the same rule is followed.
Goal: The input array contains integers representing barcodes. The length of the array is constrained as described below.
Steps:
• 1 <= barcodes.length <= 10,000
• 1 <= barcodes[i] <= 10,000
Assumptions:
• The input array contains at least one barcode.
• The solution always exists, as guaranteed by the problem statement.
• Input: Input: barcodes = [4, 4, 7, 7, 8, 8]
• Explanation: The most frequent barcode is 4, so it is placed in alternating positions. After filling the array with 4s, the remaining 7s and 8s are placed in the remaining positions. The output is [7, 4, 7, 4, 8, 8].
• Input: Input: barcodes = [1, 1, 1, 2, 2, 3]
• Explanation: The most frequent barcode is 1, so we start by placing 1 in alternating positions. Then, we place the remaining 2s and 3 in the remaining spots. The output is [1, 2, 1, 2, 1, 3].
Approach: The approach involves counting the frequency of each barcode, sorting them in descending order, and placing them in the output array by alternating between positions to ensure no adjacent barcodes are the same.
Observations:
• We need to consider the frequency of barcodes to ensure that the most frequent ones are placed first.
• The problem can be solved by sorting the barcodes by frequency and then greedily placing the most frequent barcode in available positions.
Steps:
• 1. Count the frequency of each barcode using a frequency map.
• 2. Sort the barcodes by frequency in descending order.
• 3. Place the barcodes in the output array starting from the first position and alternating between even and odd indices to ensure no adjacent barcodes are the same.
• 4. Continue placing the remaining barcodes in the remaining available positions.
Empty Inputs:
• There are no empty inputs because the array will always contain at least one barcode.
Large Inputs:
• The algorithm must efficiently handle arrays with up to 10,000 elements.
Special Values:
• If there are only one or two unique barcodes, we need to ensure the arrangement is still valid with no adjacent duplicates.
Constraints:
• The solution is guaranteed to exist as stated in the problem description.
vector<int> rearrangeBarcodes(vector<int>& b, int pos = 0) {
unordered_map<int, int> mp;
set<pair<int, int>> st;
for(int num: b) mp[num]++;
for(auto it: mp) st.insert({ it.second, it.first });
for(auto it = st.rbegin(); it != st.rend(); it++) {
for(auto cnt = 0; cnt < it->first; cnt++, pos += 2) {
if(pos >= b.size()) pos = 1;
b[pos] = it->second;
}
}
return b;
}
1 : Function Definition
vector<int> rearrangeBarcodes(vector<int>& b, int pos = 0) {
This line defines the function `rearrangeBarcodes` which takes a reference to a vector of integers `b` and an optional integer parameter `pos` (default value 0). The function aims to rearrange the elements of the vector `b` based on the barcode rearranging logic.
2 : Variable Initialization
unordered_map<int, int> mp;
An unordered map `mp` is initialized to store the frequency of each number in the barcode array `b`. The key is the barcode number, and the value is its frequency.
3 : Variable Initialization
set<pair<int, int>> st;
A set `st` is initialized to store pairs of integers, where the first element of the pair is the frequency of a barcode, and the second is the barcode number itself. The set ensures the elements are ordered by frequency in descending order.
4 : Frequency Count
for(int num: b) mp[num]++;
This loop iterates through the vector `b`, counting the frequency of each barcode and storing it in the unordered map `mp`.
5 : Sorting by Frequency
for(auto it: mp) st.insert({ it.second, it.first });
This loop iterates over the map `mp` and inserts each barcode along with its frequency as a pair into the set `st`. The set will automatically order the pairs by the frequency in descending order.
6 : Descending Loop
for(auto it = st.rbegin(); it != st.rend(); it++) {
This loop iterates through the set `st` in reverse order (from the most frequent to the least frequent barcode), using a reverse iterator `rbegin()` and `rend()`.
7 : Placement Logic
for(auto cnt = 0; cnt < it->first; cnt++, pos += 2) {
This inner loop places the barcodes into the vector `b`. It runs for the frequency of the current barcode (`it->first`). It also increments `pos` by 2 to ensure that the barcodes are placed in alternate positions.
8 : Edge Case Handling
if(pos >= b.size()) pos = 1;
This condition checks if `pos` exceeds the size of the vector `b`. If it does, `pos` is reset to 1, ensuring that the placement continues from the second position (odd indices).
9 : Barcode Assignment
b[pos] = it->second;
The barcode `it->second` (which is the barcode number) is assigned to the position `pos` in the vector `b`.
10 : Return Statement
return b;
The function returns the modified vector `b` with the rearranged barcodes.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is O(n log n) due to the sorting step, followed by a linear scan to fill the array.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) because we use additional space for counting the frequency of each barcode.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus