Leetcode 1043: Partition Array for Maximum Sum
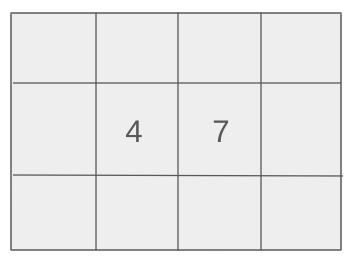
Given an array of integers, partition the array into contiguous subarrays where each subarray has a length of at most k. After partitioning, transform each subarray such that all its elements are replaced by the maximum element of that subarray. Your goal is to return the maximum sum of the transformed array.
Problem
Approach
Steps
Complexity
Input: You are given an integer array arr and an integer k, the maximum length of a subarray.
Example: Input: arr = [2, 7, 3, 4, 8], k = 3
Constraints:
• 1 <= arr.length <= 500
• 0 <= arr[i] <= 10^9
• 1 <= k <= arr.length
Output: Return the largest sum of the given array after partitioning, where each subarray is replaced by its maximum value.
Example: Output: 30
Constraints:
• The answer is guaranteed to fit within a 32-bit integer.
Goal: The goal is to find the largest possible sum after partitioning the array into subarrays, each with a maximum length of k, and transforming the elements of each subarray by replacing them with the maximum value of that subarray.
Steps:
• 1. Use dynamic programming to store the results of subproblems.
• 2. For each possible partition point, calculate the sum of the maximum values of the subarrays formed.
• 3. Apply a greedy approach to maximize the sum by selecting the optimal partitioning of the array.
Goal: The problem is constrained by the size of the array and the length of subarrays that can be formed.
Steps:
• 1 <= arr.length <= 500
• 0 <= arr[i] <= 10^9
• 1 <= k <= arr.length
Assumptions:
• The array can have at most 500 elements.
• The maximum value for each element is 10^9.
• Input: Input: arr = [2, 7, 3, 4, 8], k = 3
• Explanation: In this case, the array can be partitioned as [2, 7, 3] and [4, 8]. After replacing each subarray with its maximum value, the transformed array becomes [7, 8]. The sum of this transformed array is 30.
• Input: Input: arr = [1, 4, 1, 5, 7, 3, 6, 1, 9, 9, 3], k = 4
• Explanation: Here, we can partition the array into subarrays such as [1, 4, 1, 5], [7, 3, 6, 1], and [9, 9, 3]. The transformed array becomes [5, 7, 9], and the maximum sum is 83.
Approach: The problem can be solved efficiently using dynamic programming where we try to partition the array at different points and calculate the sum of the maximum values of the subarrays. The key idea is to iteratively calculate the maximum sum by considering all possible partitions of the array.
Observations:
• This problem is a dynamic programming problem where we need to maximize the sum by dividing the array into subarrays and transforming them.
• The dynamic programming solution will help us avoid redundant calculations by storing intermediate results for subproblems.
Steps:
• 1. Create a dp array where dp[i] represents the maximum sum for the first i elements.
• 2. Iterate over the array and try partitioning it at every possible point up to k.
• 3. For each partition, calculate the maximum sum by considering the maximum element in each subarray formed.
Empty Inputs:
• The array will always have at least one element, so no need to handle empty arrays.
Large Inputs:
• For larger arrays (up to 500 elements), the dynamic programming approach should be efficient enough.
Special Values:
• If k equals the length of the array, then the whole array is a single subarray.
Constraints:
• The array will always contain at least one element and will never exceed 500 elements.
vector<int> memo;
vector<int> arr;
int k;
int dp(int i) {
if(i == arr.size()) return 0;
if(memo[i] != -1) return memo[i];
int res = 0, mx = 0;
for(int j = i; j < min((int)arr.size(), i + k); j++) {
mx = max(mx, arr[j]);
res = max(res, mx * (j - i + 1) + dp(j + 1));
}
return memo[i] = res;
}
int maxSumAfterPartitioning(vector<int>& arr, int k) {
this->arr = arr;
this->k = k;
memo.resize(arr.size(), -1);
return dp(0);
}
1 : Variable Declaration
vector<int> memo;
Declare a memoization vector 'memo' to store the results of subproblems and avoid redundant calculations.
2 : Variable Declaration
vector<int> arr;
Declare a vector 'arr' to hold the input array.
3 : Variable Declaration
int k;
Declare an integer 'k' to store the maximum length of subarrays that can be created during the partitioning process.
4 : Function Definition
int dp(int i) {
Define the recursive function 'dp' that solves the problem for subarrays starting at index 'i'.
5 : Base Case
if(i == arr.size()) return 0;
If the index 'i' has reached the end of the array, return 0 since no further partitions can be made.
6 : Memoization
if(memo[i] != -1) return memo[i];
Check if the subproblem for index 'i' has been solved before. If so, return the stored result from the 'memo' vector.
7 : Variable Initialization
int res = 0, mx = 0;
Initialize the variables 'res' to store the maximum result and 'mx' to store the maximum value of the current subarray.
8 : Loop Through Subarrays
for(int j = i; j < min((int)arr.size(), i + k); j++) {
Start a loop to explore all possible subarrays starting at index 'i' with a length of at most 'k'.
9 : Update Maximum Value
mx = max(mx, arr[j]);
Update 'mx' to the maximum value between the current element 'arr[j]' and the previous maximum 'mx'.
10 : Calculate Result
res = max(res, mx * (j - i + 1) + dp(j + 1));
For each subarray, calculate the potential result by multiplying the maximum value 'mx' by the subarray length and adding the result of the next subarray (recursively).
11 : Memoization Update
return memo[i] = res;
Store the result of the current subproblem in the 'memo' vector and return it.
12 : Function Definition
int maxSumAfterPartitioning(vector<int>& arr, int k) {
Define the function 'maxSumAfterPartitioning' which serves as the entry point to initialize variables and call the recursive 'dp' function.
13 : Variable Initialization
this->arr = arr;
Assign the input array 'arr' to the class variable for use in the recursive function.
14 : Variable Initialization
this->k = k;
Assign the value of 'k' (maximum subarray length) to the class variable.
15 : Memoization Initialization
memo.resize(arr.size(), -1);
Resize the 'memo' vector to match the size of the input array and initialize all values to -1, indicating that no subproblems have been solved yet.
16 : Return Result
return dp(0);
Call the 'dp' function starting from index 0 to compute and return the maximum sum after partitioning the array.
Best Case: O(n)
Average Case: O(n * k)
Worst Case: O(n * k)
Description: The time complexity is O(n * k) because for each element, we check up to k previous elements.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) for storing the dp array.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus