Leetcode 1014: Best Sightseeing Pair
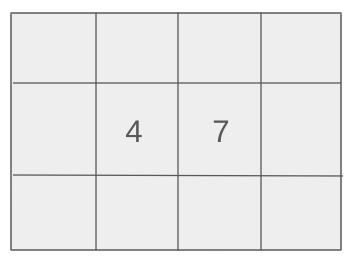
You are given an array where each element represents the value of a sightseeing spot. The distance between two sightseeing spots is the difference in their indices. The score of a pair of sightseeing spots i and j (where i < j) is calculated as values[i] + values[j] + (i - j), which includes the values of both spots and subtracts the distance between them. Your task is to find the maximum score of any pair of sightseeing spots.
Problem
Approach
Steps
Complexity
Input: The input consists of an array of integers, where each integer represents the value of a sightseeing spot.
Example: values = [5, 3, 6, 2, 4]
Constraints:
• 2 <= values.length <= 5 * 10^4
• 1 <= values[i] <= 1000
Output: The output should be a single integer representing the maximum score of any pair of sightseeing spots.
Example: Output: 8
Constraints:
• The result should be an integer representing the maximum score.
Goal: The goal is to find the pair of sightseeing spots that maximizes the score, which involves considering both the values and the distance between the spots.
Steps:
• Iterate through the array and for each spot, compute the score with all previous spots while keeping track of the maximum score.
• Maintain a running value for the maximum score by subtracting the distance between the spots.
Goal: Ensure the solution is efficient enough to handle the input size within the given constraints.
Steps:
• The number of sightseeing spots can be up to 50,000, so the solution must be optimized for time complexity.
• Each value in the array is between 1 and 1000.
Assumptions:
• The input will always have at least two sightseeing spots.
• Input: Input: values = [5, 3, 6, 2, 4]
• Explanation: In this case, the maximum score occurs between the first and third spots: 5 + 6 + (0 - 2) = 8.
• Input: Input: values = [1, 2]
• Explanation: For this example, the score is 1 + 2 + (0 - 1) = 2, which is the maximum score.
Approach: The optimal approach is to iterate through the array, maintaining a running maximum score based on the current spot and the previous ones. This can be done efficiently using a greedy approach.
Observations:
• We need to maximize the score, which involves both the values of the sightseeing spots and the distance between them.
• By iterating through the array while keeping track of the maximum score at each step, we can find the solution in linear time.
Steps:
• 1. Initialize a variable to store the maximum score (res).
• 2. Initialize a variable (cur) to keep track of the best score calculated so far as we iterate through the list.
• 3. Iterate through the array, updating res with the maximum score at each step.
• 4. Update cur with the best possible score for the next iteration by considering the value at the current spot and subtracting the distance from the previous spot.
Empty Inputs:
• The problem guarantees that the input will always have at least two values.
Large Inputs:
• The solution should be efficient enough to handle up to 50,000 spots in linear time.
Special Values:
• If all values are the same, the solution should still correctly calculate the maximum score based on the distances.
Constraints:
• The number of spots is at least 2, ensuring there is always a valid pair.
int maxScoreSightseeingPair(vector<int>& val) {
int res = 0, cur = 0;
for(int x : val) {
res = max(res, cur + x);
cur = max(cur, x) - 1;
}
return res;
}
1 : Function Declaration
int maxScoreSightseeingPair(vector<int>& val) {
Declares the function `maxScoreSightseeingPair` which takes a vector of integers `val` representing the values of sightseeing spots, and returns an integer representing the maximum score of a sightseeing pair.
2 : Initialization
int res = 0, cur = 0;
Sets `res` to 0, as initially no score has been calculated, and `cur` to 0, which will hold the current potential score during the iteration.
3 : Loop Through Values
for(int x : val) {
Iterates over each value `x` in the `val` vector representing the value of a sightseeing spot.
4 : Update Result
res = max(res, cur + x);
Updates the maximum score `res` by comparing the current score `cur + x` (the sum of the current potential score and the current value) with the previous maximum score.
5 : Update Current Score
cur = max(cur, x) - 1;
Updates the current potential score `cur` by comparing it with the value `x`. The current score is reduced by 1 as part of the problem's condition (since the second point must be after the first).
6 : Return Statement
return res;
Returns the maximum score of a sightseeing pair, which is the result of evaluating all possible pairs.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The solution iterates through the array once, leading to a time complexity of O(n), where n is the number of sightseeing spots.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) since the solution only uses a few variables to track the maximum score and the current best score.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus