Leetcode 1006: Clumsy Factorial
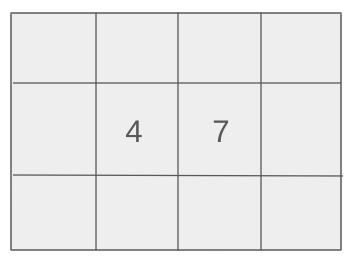
Given a positive integer n, compute the ‘clumsy factorial’ of n. A clumsy factorial is computed using a series of operations (multiplication, division, addition, subtraction) in a fixed order applied to the integers from n down to 1. The operations follow this order: multiplication ‘*’, division ‘/’, addition ‘+’, subtraction ‘-’, and are repeated cyclically. Division is performed as floor division, and multiplication and division are processed left to right before addition and subtraction.
Problem
Approach
Steps
Complexity
Input: The input consists of a single integer n (1 <= n <= 10^4).
Example: n = 6
Constraints:
• 1 <= n <= 10^4
Output: Return the computed clumsy factorial as an integer.
Example: Output: 7
Constraints:
• The output will be a single integer result of the clumsy factorial.
Goal: The goal is to compute the clumsy factorial of n by following the predefined rotation of operations and respecting the order of operations.
Steps:
• Start with the first integer, n.
• Apply the operations (multiplication, division, addition, subtraction) in a cyclic order while considering left-to-right precedence for multiplication and division.
• Use floor division for all division operations.
• Keep track of the intermediate results in a stack to handle the addition and subtraction steps.
• Finally, compute the sum of the results and return the value.
Goal: Ensure that the solution can handle large values of n efficiently.
Steps:
• The solution should be efficient enough to handle n up to 10,000.
Assumptions:
• The input n is always a positive integer.
• The solution needs to follow the order of operations as described.
• Input: Input: n = 4
• Explanation: The clumsy factorial of 4 is computed as follows: 4 * 3 / 2 + 1 = 7.
• Input: Input: n = 6
• Explanation: The clumsy factorial of 6 is computed as follows: 6 * 5 / 4 + 3 - 2 * 1 = 7.
Approach: To solve the problem, we will simulate the operations as described, using a stack to handle the addition and subtraction operations and performing multiplication and division left-to-right as needed.
Observations:
• The problem follows a fixed order of operations and can be approached step by step.
• Since multiplication and division take precedence, we will perform those first and use a stack for the addition and subtraction steps.
Steps:
• Initialize the first number as the result.
• Iterate over the range from n-1 to 1, applying the operations in the cyclic order (multiplication, division, addition, subtraction).
• Use a stack to hold intermediate results for addition and subtraction operations.
• Return the final computed value after performing all operations.
Empty Inputs:
• The input will always contain a valid integer n, so there are no empty inputs.
Large Inputs:
• The solution should efficiently handle the maximum constraint of n = 10,000.
Special Values:
• If n is 1, the clumsy factorial is simply 1.
Constraints:
• The solution must handle values of n efficiently up to the maximum constraint of 10,000.
int clumsy(int n) {
int ans = n;
int j = n - 1;
vector<int> stk;
for(int i = 1; i < n; i++) {
if(i % 4 == 1) ans *= j--;
else if(i % 4 == 2) ans /= j--;
else {
stk.push_back(ans);
if(i % 4 == 3) ans = j--;
else ans = (-1 * j--);
}
}
int sum = 0;
for(int i = 0; i < stk.size(); i++)
sum += stk[i];
return sum + ans;
}
1 : Function Definition
int clumsy(int n) {
Defines the function `clumsy`, which takes an integer `n` and returns its clumsy factorial based on custom arithmetic operations.
2 : Initialization
int ans = n;
Initializes `ans` to `n`, which will hold the result of the clumsy factorial calculation.
3 : Pointer Setup
int j = n - 1;
Initializes `j` as `n - 1` to use as a pointer for the calculation of intermediate values.
4 : Stack Initialization
vector<int> stk;
Creates a vector `stk` to temporarily store intermediate results for the clumsy factorial.
5 : Loop Start
for(int i = 1; i < n; i++) {
Starts a loop from 1 to `n - 1` to apply the custom operations based on the current step.
6 : Multiplication Step
if(i % 4 == 1) ans *= j--;
If `i % 4 == 1`, multiply `ans` by `j` and then decrement `j`.
7 : Division Step
else if(i % 4 == 2) ans /= j--;
If `i % 4 == 2`, divide `ans` by `j` and then decrement `j`.
8 : Stack Push Operation
stk.push_back(ans);
Pushes the current value of `ans` to the stack.
9 : Reset/Update Operation
if(i % 4 == 3) ans = j--;
If `i % 4 == 3`, set `ans` to `j` and then decrement `j`.
10 : Reset/Negative Operation
else ans = (-1 * j--);
For other values of `i % 4`, set `ans` to the negative value of `j` and then decrement `j`.
11 : Sum Initialization
int sum = 0;
Initializes `sum` to accumulate the values from the stack.
12 : Summing Stack Values
for(int i = 0; i < stk.size(); i++)
Starts a loop to sum the values in the stack.
13 : Accumulate Stack Values
sum += stk[i];
Adds each element in the stack to the `sum`.
14 : Return Statement
return sum + ans;
Returns the final result, which is the sum of the stack values plus the last calculated `ans`.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) since we only need to iterate through the numbers and perform constant-time operations for each one.
Best Case: O(1)
Worst Case: O(n)
Description: The space complexity is O(n) due to the stack used to store intermediate results.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus